用c语言判断一个数是否为回文数
时间: 2024-11-25 19:33:22 浏览: 30
在C语言中,判断一个整数是否为回文数可以通过比较数字前后对称的部分是否相同来实现。以下是一个简单的算法步骤:
1. 将输入的整数转换为字符串,因为可以直接从字符串两端开始比较字符,而不需要关心进位。
2. 创建两个指针,一个指向字符串的开头(高位),另一个指向结尾(低位)。
3. 比较这两个位置上的字符是否相等。如果相等,则继续比较下一个字符;如果不相等,则该数不是回文数。
4. 当两个指针相遇或交叉时,说明已经检查了整个字符串的一半,如果没有发现不匹配的字符,那么这个数就是回文数。
下面是一个示例程序,实现了上述逻辑:
```c
#include <stdio.h>
#include <string.h>
int isPalindrome(int num) {
// Convert the number to a string
char str[100];
sprintf(str, "%d", num);
// Create pointers for start and end of the string
int start = 0;
int end = strlen(str) - 1;
while (start < end) {
if (str[start] != str[end]) {
return 0; // Not a palindrome
}
start++;
end--;
}
return 1; // It's a palindrome
}
int main() {
int num;
printf("Enter a number: ");
scanf("%d", &num);
if (isPalindrome(num)) {
printf("%d is a palindrome.\n", num);
} else {
printf("%d is not a palindrome.\n", num);
}
return 0;
}
```
阅读全文
相关推荐
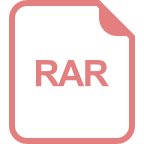
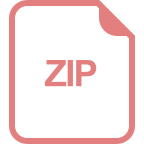















