为了便于操作和观察结果,用一个 程序为“引子”,先后fork( )两个子进程,SERVER和CLIENT,进行通信。SERVER端建立一个Key为75的消息队列,等待其他进程发来的消息。当遇到类型为1的消息,则作为结束信号,取消该队列,并退出SERVER 。SERVER每接收到一个消息后显示一句“(server)received”。CLIENT端使用Key为75的消息队列,先后发送类型从10到1的消息,然后退出。最后的一个消息,既是 SERVER端需要的结束信号。CLIENT每发送一条消息后显示一句“(client)sent”。父进程在 SERVER和 CLIENT均退出后结束。
时间: 2024-02-19 18:57:15 浏览: 32
好的,您的问题是如何实现一个程序,使用消息队列进行进程间通信,其中包括一个SERVER进程和一个CLIENT进程,SERVER进程建立一个Key为75的消息队列等待其他进程发来消息,遇到类型为1的消息则取消该队列并退出,每接收到一条消息显示一句“(server)received”,CLIENT进程使用Key为75的消息队列,先后发送类型从10到1的消息,最后发送一个类型为1的消息作为结束信号,每发送一条消息后显示一句“(client)sent”,父进程在SERVER和CLIENT均退出后结束。
这个问题可以用C语言来实现,以下是一个简单的实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <unistd.h>
#define MSG_TYPE_STOP 1
struct msgbuf {
long mtype;
char mtext[256];
};
void server(int msqid) {
struct msgbuf buf;
while (1) {
if (msgrcv(msqid, &buf, sizeof(buf.mtext), 0, 0) == -1) {
perror("msgrcv failed");
exit(EXIT_FAILURE);
}
printf("(server)received\n");
if (buf.mtype == MSG_TYPE_STOP) {
if (msgctl(msqid, IPC_RMID, NULL) == -1) {
perror("msgctl (remove) failed");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
}
}
void client(int msqid) {
struct msgbuf buf;
for (int i = 10; i >= 1; i--) {
buf.mtype = i;
sprintf(buf.mtext, "message %d from client", i);
if (msgsnd(msqid, &buf, sizeof(buf.mtext), 0) == -1) {
perror("msgsnd failed");
exit(EXIT_FAILURE);
}
printf("(client)sent\n");
}
buf.mtype = MSG_TYPE_STOP;
sprintf(buf.mtext, "stop message from client");
if (msgsnd(msqid, &buf, sizeof(buf.mtext), 0) == -1) {
perror("msgsnd failed");
exit(EXIT_FAILURE);
}
printf("(client)sent\n");
}
int main() {
int msqid;
key_t key = 75;
if ((msqid = msgget(key, 0666 | IPC_CREAT)) == -1) {
perror("msgget failed");
exit(EXIT_FAILURE);
}
pid_t server_pid, client_pid;
if ((server_pid = fork()) == -1) {
perror("fork failed");
exit(EXIT_FAILURE);
} else if (server_pid == 0) {
server(msqid);
} else {
if ((client_pid = fork()) == -1) {
perror("fork failed");
exit(EXIT_FAILURE);
} else if (client_pid == 0) {
client(msqid);
} else {
int status;
waitpid(server_pid, &status, 0);
waitpid(client_pid, &status, 0);
if (msgctl(msqid, IPC_RMID, NULL) == -1) {
perror("msgctl (remove) failed");
exit(EXIT_FAILURE);
}
printf("(parent)exiting\n");
exit(EXIT_SUCCESS);
}
}
}
```
在这个代码中,我们先调用`msgget`函数创建一个Key为75的消息队列,在主进程中使用`fork`函数创建一个SERVER进程和一个CLIENT进程,分别执行`server`和`client`函数。SERVER进程使用`msgrcv`函数从消息队列中接收消息,接收到的消息类型为0,表示接收任意类型的消息,同时将消息类型和消息内容打印出来,如果接收到类型为1的消息,则执行`msgctl`函数删除消息队列,并退出进程。CLIENT进程使用`msgsnd`函数向消息队列中发送10条类型从10到1的消息和一条类型为1的消息作为结束信号,并将每条消息的内容打印出来。父进程使用`waitpid`函数等待SERVER和CLIENT进程退出,然后执行`msgctl`函数删除消息队列,并打印一条退出信息。
这个程序实现了两个进程间使用消息队列进行通信的功能,可以通过编译运行测试。注意在测试时要先运行该程序,然后再在另一个终端中使用`ipcs -q`命令查看消息队列是否存在,使用`ipcrm -q 75`命令删除消息队列。
相关推荐
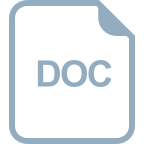
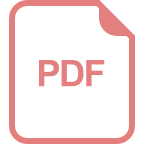
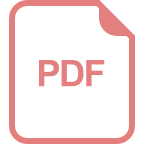














