连接http传文件捕获异常try catch
时间: 2023-11-23 22:45:48 浏览: 128
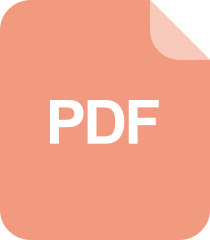
简单谈谈java的异常处理(Try Catch Finally)
在Java中,使用URLConnection可以创建一个HTTP连接并发送请求。以下是一个使用URLConnection从http传输文件的示例,并在传输过程中捕获异常:
```java
import java.io.*;
import java.net.*;
public class HttpFileUploader {
public static void main(String[] args) {
String url = "http://example.com/upload";
String fileToUpload = "/path/to/file.jpg";
try {
URL fileUrl = new URL(fileToUpload);
URLConnection connection = new URL(url).openConnection();
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type", "application/octet-stream");
connection.setRequestProperty("Content-Disposition", "attachment; filename=\"" + fileUrl.getFile() + "\"");
OutputStream out = connection.getOutputStream();
InputStream in = new FileInputStream(new File(fileToUpload));
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
in.close();
out.flush();
out.close();
// Handle response
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line = null;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们使用URLConnection打开一个HTTP连接,并设置请求属性。我们还打开了一个输入流来读取文件,并使用输出流将文件上传到服务器。最后,我们使用一个缓冲读取器来读取服务器的响应。如果在传输文件过程中出现任何异常,就会被try-catch块捕获并打印出堆栈跟踪信息。
阅读全文
相关推荐
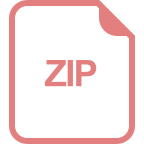
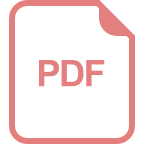















