编写js编写 做一个建议的停车场使用数据记录仪 此程序能够监听命令行的输入,命令行可以输入三类命令:(下列命令中的数值均为举例,实际使用中不会限定数值) 1:checkin -t=225959 -n=AT4257 2:checkout -t=233
时间: 2024-05-09 20:21:02 浏览: 14
959 -n=AT4257 3:report
其中,checkin命令表示车辆进入停车场,需要记录时间和车牌号;checkout命令表示车辆离开停车场,需要记录时间和车牌号,并计算停车时间和费用;report命令表示生成停车场使用报告,包括停车总量、停车总时长、总收入等信息。
以下是实现代码:
```javascript
const readline = require('readline');
// 定义停车场类
class ParkingLot {
constructor() {
this.records = {}; // 停车记录
this.totalCount = 0; // 停车总量
this.totalTime = 0; // 停车总时长
this.totalIncome = 0; // 总收入
}
checkin(time, plateNumber) {
if (this.records[plateNumber]) {
console.log(`${plateNumber}已经在停车场内`);
return;
}
this.records[plateNumber] = { checkinTime: time };
this.totalCount++;
console.log(`${plateNumber}进入停车场,时间为${time}`);
}
checkout(time, plateNumber) {
if (!this.records[plateNumber]) {
console.log(`${plateNumber}不在停车场内`);
return;
}
const record = this.records[plateNumber];
const checkinTime = record.checkinTime;
const duration = time - checkinTime; // 停车时长
const cost = Math.ceil(duration / 1000 / 60) * 2; // 停车费用
delete this.records[plateNumber];
this.totalTime += duration;
this.totalIncome += cost;
console.log(`${plateNumber}离开停车场,停车时长为${duration}ms,停车费用为${cost}元`);
}
report() {
console.log(`停车总量:${this.totalCount}`);
console.log(`停车总时长:${this.totalTime}ms`);
console.log(`总收入:${this.totalIncome}元`);
}
}
// 初始化停车场
const parkingLot = new ParkingLot();
// 监听命令行输入
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.on('line', (input) => {
const [command, options] = input.split(' ');
const args = {};
options.split('-').slice(1).forEach((option) => {
const [key, value] = option.split('=');
args[key] = value;
});
switch (command) {
case 'checkin':
parkingLot.checkin(args.t, args.n);
break;
case 'checkout':
parkingLot.checkout(args.t, args.n);
break;
case 'report':
parkingLot.report();
break;
default:
console.log('无效命令');
}
});
```
使用方法:
在命令行中运行以上代码,然后输入命令即可,例如:
```
checkin -t=225959 -n=AT4257
checkout -t=233959 -n=AT4257
report
```
相关推荐
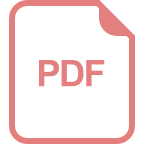
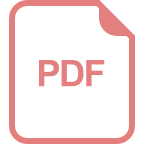














