C语言用指针实现对给定字符串查找给定的子串并输出第一个子串所在的位置
时间: 2024-05-07 17:21:36 浏览: 55
可以使用指针和字符串库函数来实现这个功能。以下是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100], substr[100];
printf("Enter a string: ");
fgets(str, 100, stdin);
printf("Enter a substring to search for: ");
fgets(substr, 100, stdin);
// Remove newline characters from input strings
strtok(str, "\n");
strtok(substr, "\n");
int pos = -1; // Initialize position to -1 (not found)
char *ptr = strstr(str, substr); // Find first occurrence of substring
if (ptr != NULL) {
pos = ptr - str; // Calculate position of substring
}
printf("Substring found at position %d\n", pos);
return 0;
}
```
首先,我们使用 `fgets()` 函数从标准输入中读取字符串和子串。然后,我们使用 `strtok()` 函数删除字符串中的换行符。接下来,我们使用 `strstr()` 函数在字符串中查找子串。如果找到了子串,则计算子串在字符串中的位置(即指针的差值),并将其存储在变量 `pos` 中。最后,我们输出子串的位置。如果未找到子串,则 `pos` 仍为 -1。
阅读全文
相关推荐
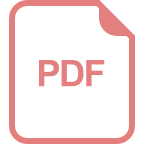
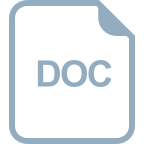

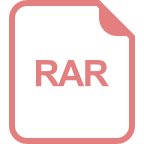
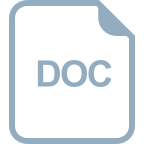










