用c语言编写一个程序,从键盘输入一个年份,判断并输出它是闰年还是平年
时间: 2023-05-14 08:04:42 浏览: 195
好的,以下是用 C 语言编写的程序,可以判断输入的年份是闰年还是平年:
```c
#include <stdio.h>
int main() {
int year;
printf("请输入一个年份:");
scanf("%d", &year);
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
printf("%d年是闰年\n", year);
} else {
printf("%d年是平年\n", year);
}
return 0;
}
```
这个程序会先从键盘读入一个年份,然后通过判断这个年份是否能被 4 整除但不能被 100 整除,或者能被 400 整除来判断它是闰年还是平年。如果是闰年,就输出“是闰年”,否则输出“是平年”。
相关问题
编写一个c语言程序从键盘输入一个日期,要求计算该日期是这一年的第几天
编写C语言程序来计算给定日期是一年中的第几天,通常涉及到闰年的判断。以下是简单的步骤和代码示例:
1. 定义一个函数,接受年份、月份和日期作为参数。
2. 判断是否是闰年。闰年规则:能被4整除的年份是闰年,但是能被100整除的不是,除非同时也能被400整除。
3. 计算每个月的天数,考虑二月特殊情况(平年28天,闰年29天)。
4. 根据给定的日期逐月累加天数。
下面是一个基础的代码示例:
```c
#include <stdio.h>
// Function to check if a year is leap
int is_leap(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// Calculate days in each month
int days_in_month(int month, int year) {
switch(month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
return 31;
case 4: case 6: case 9: case 11:
return 30;
case 2:
return is_leap(year) ? 29 : 28;
default:
return -1; // Invalid month
}
}
// Main function
int main() {
int year, month, day;
printf("Enter the date (YYYY-MM-DD): ");
scanf("%d-%d-%d", &year, &month, &day);
// Ensure input is valid
if(month > 12 || day > days_in_month(month, year)) {
printf("Invalid date.\n");
return 1;
}
int total_days = 0;
for(int i = 1; i < month; ++i) {
total_days += days_in_month(i, year);
}
total_days += day;
printf("The given date is the %dth day of %d.\n", total_days, year);
return 0;
}
```
19.(简答题)-|||-天数判断。-|||-题目描述-|||-题目内容:编写程序,从键盘上输入年份和月份,计算并输出这一年的这一月共有多少天。输入样例:2019-3输出样例:31用c语言
在C语言中,判断一年中某个月份的天数,我们需要考虑闰年和平年的规则。以下是简单的步骤:
1. 首先检查是否为闰年:
- 如果年份能被4整除但不能被100整除,或者可以被400整除,则是闰年。
2. 然后根据月份确定天数:
- 一月和二月:一般情况下一月有31天,二月有28天(闰年则有29天)。
- 三月至十二月:都是30天或31天,具体取决于月份。
以下是示例代码片段:
```c
#include <stdio.h>
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int main() {
int year, month;
// 输入年份和月份
printf("请输入年份(如2019)和月份(如3): ");
scanf("%d-%d", &year, &month);
// 检查是否为闰年
if (is_leap_year(year)) {
if (month == 2) {
printf("%d-%d: %d\n", year, month, 29); // 闰年的2月有29天
} else if (month <= 2) {
printf("%d-%d: %d\n", year, month, 31); // 1月、2月有31天
} else {
printf("%d-%d: %d\n", year, month, 30); // 其他非2月的月份有30天
}
} else { // 平年
if (month == 2) {
printf("%d-%d: %d\n", year, month, 28); // 平年的2月有28天
} else {
printf("%d-%d: %d\n", year, month, (month > 7) ? 30 : 31); // 根据月份判断其他月份的天数
}
}
return 0;
}
```
阅读全文
相关推荐
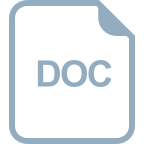
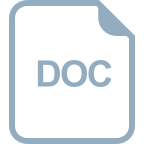
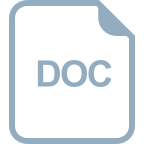
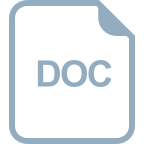
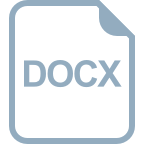
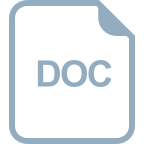
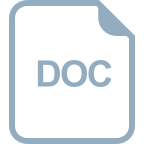
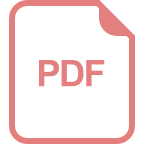
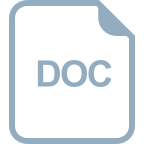


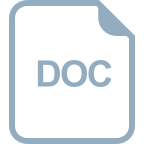
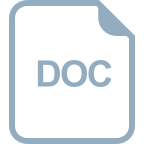
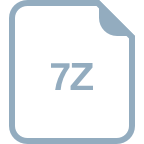
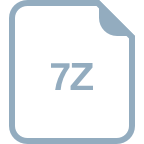