如何根据results.cs$文件中的数据来创建和展示F1的图表?
时间: 2024-10-06 15:00:34 浏览: 24
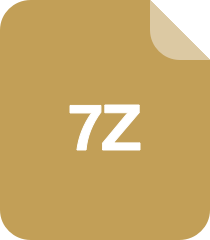
jmeter.results.shanhe.me.xsl文件
为了根据`results.cs`文件中的数据创建和展示F1分数的图表,你需要遵循以下步骤:
1. **加载数据**:
- 首先,你需要读取`results.cs`文件并解析其中包含的F1分数数据。这通常涉及到使用文本文件操作、CSV库(如C#的`System.IO`或第三方库如CsvHelper)或直接从数据库获取。
```csharp
string[] lines = File.ReadAllLines("results.cs");
List<(int index, double f1Score)> dataPoints = new List<(int, double)>();
foreach (string line in lines)
{
string[] values = line.Split(',');
if (values.Length >= 2)
{
int index = int.Parse(values[0]);
double f1 = double.Parse(values[1]);
dataPoints.Add((index, f1));
}
}
```
2. **数据预处理**:
确保数据清理完成,比如检查是否有缺失值或异常值,并对需要的数值(如F1分数)进行适当归一化。
3. **选择图表库**:
C#有很多图表库可以使用,例如Microsoft Chart Controls for .NET Framework、OxyPlot、LiveCharts等。这里以OxyPlot为例:
```csharp
using OxyPlot;
using OxyPlot.Axes;
using OxyPlot.Series;
var plotModel = new PlotModel();
plotModel.Title = "F1 Score Over Time";
// 创建日期轴
DateTimeAxis dateTimeAxis = new DateTimeAxis { Position = AxisPosition.Bottom };
dateTimeAxis.LabelFormatter = val => val.ToString("yyyy-MM-dd");
// 创建数值轴
LinearAxis f1Axis = new LinearAxis { Position = AxisPosition.Left, Title = "F1 Score" };
// 创建线形系列
var series = new LineSeries();
series.Points.AddRange(dataPoints.Select(point => new DataPoint(dateTimeAxis.ToDouble(point.index), point.f1)));
plotModel.Axes.Add(dateTimeAxis);
plotModel.Axes.Add(f1Axis);
plotModel.Series.Add(series);
// 显示图表
var plotView = new OxyPlot.WindowsForms.PlotView();
plotView.Model = plotModel;
// 将plotView添加到窗体或用户界面控件上
```
4. **显示图表**:
如果是在WPF应用中,你会将`plotView`添加到窗口;如果是控制台应用程序,则可能需要额外的UI框架(如ConsoleChartProvider)来显示图表。
阅读全文
相关推荐
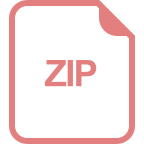
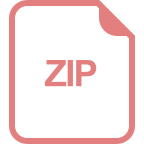
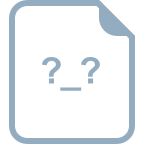
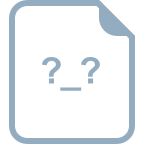
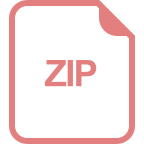
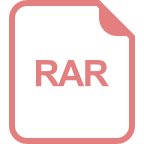
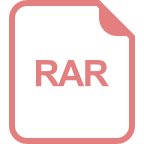
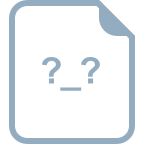
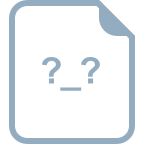
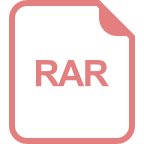
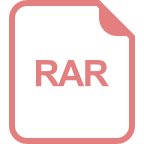
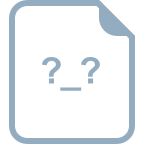
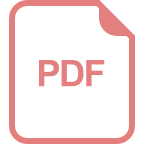
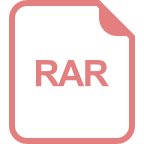
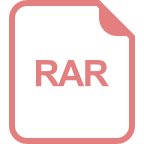
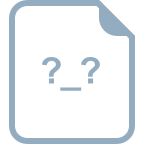