用java设计和编写一个简易的带小数点的计算器:实现计算器的界面;实现计算按钮不同的计算功能;显示计算器按钮运算后的结果。
时间: 2024-02-19 22:58:05 浏览: 46
好的,我会尽力回答你的问题。
首先,为了实现计算器的界面,我们可以使用Java的Swing库。我们可以使用JFrame作为主窗口,并在其中添加各种按钮和文本框。以下是一个示例代码:
```java
import javax.swing.*;
public class CalculatorGUI {
public static void main(String[] args) {
JFrame frame = new JFrame("Calculator");
frame.setSize(300, 400); // 设置窗口大小
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // 设置关闭窗口时的操作
// 添加文本框
JTextField textField = new JTextField();
textField.setBounds(10, 10, 260, 40);
frame.add(textField);
// 添加数字按钮
int x = 10, y = 60, width = 60, height = 60;
for (int i = 0; i < 10; i++) {
JButton button = new JButton(String.valueOf(i));
button.setBounds(x, y, width, height);
frame.add(button);
x += 70;
if (i % 3 == 2) {
x = 10;
y += 70;
}
}
// 添加小数点
JButton dotButton = new JButton(".");
dotButton.setBounds(80, 290, width, height);
frame.add(dotButton);
// 添加计算按钮
JButton addButton = new JButton("+");
addButton.setBounds(220, 60, width, height);
frame.add(addButton);
JButton minusButton = new JButton("-");
minusButton.setBounds(220, 130, width, height);
frame.add(minusButton);
JButton multiplyButton = new JButton("*");
multiplyButton.setBounds(220, 200, width, height);
frame.add(multiplyButton);
JButton divideButton = new JButton("/");
divideButton.setBounds(220, 270, width, height);
frame.add(divideButton);
// 添加等号按钮
JButton equalButton = new JButton("=");
equalButton.setBounds(150, 290, width, height);
frame.add(equalButton);
frame.setLayout(null);
frame.setVisible(true);
}
}
```
接下来,我们需要实现计算按钮不同的计算功能。我们可以使用Java的计算表达式库来实现。以下是一个示例代码:
```java
import javax.script.ScriptEngine;
import javax.script.ScriptEngineManager;
import javax.script.ScriptException;
import javax.swing.*;
public class CalculatorGUI {
public static void main(String[] args) {
JFrame frame = new JFrame("Calculator");
frame.setSize(300, 400); // 设置窗口大小
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // 设置关闭窗口时的操作
// 添加文本框
JTextField textField = new JTextField();
textField.setBounds(10, 10, 260, 40);
frame.add(textField);
// 添加数字按钮
int x = 10, y = 60, width = 60, height = 60;
for (int i = 0; i < 10; i++) {
JButton button = new JButton(String.valueOf(i));
button.setBounds(x, y, width, height);
button.addActionListener(e -> {
textField.setText(textField.getText() + i);
});
frame.add(button);
x += 70;
if (i % 3 == 2) {
x = 10;
y += 70;
}
}
// 添加小数点
JButton dotButton = new JButton(".");
dotButton.setBounds(80, 290, width, height);
dotButton.addActionListener(e -> {
textField.setText(textField.getText() + ".");
});
frame.add(dotButton);
// 添加计算按钮
JButton addButton = new JButton("+");
addButton.setBounds(220, 60, width, height);
addButton.addActionListener(e -> {
String expression = textField.getText();
if (!expression.isEmpty()) {
textField.setText("");
try {
ScriptEngineManager manager = new ScriptEngineManager();
ScriptEngine engine = manager.getEngineByName("js");
Object result = engine.eval(expression);
textField.setText(result.toString());
} catch (ScriptException ex) {
JOptionPane.showMessageDialog(frame, "Invalid expression", "Error", JOptionPane.ERROR_MESSAGE);
}
}
});
frame.add(addButton);
JButton minusButton = new JButton("-");
minusButton.setBounds(220, 130, width, height);
minusButton.addActionListener(e -> {
String expression = textField.getText();
if (!expression.isEmpty()) {
textField.setText("");
try {
ScriptEngineManager manager = new ScriptEngineManager();
ScriptEngine engine = manager.getEngineByName("js");
Object result = engine.eval(expression);
textField.setText(result.toString());
} catch (ScriptException ex) {
JOptionPane.showMessageDialog(frame, "Invalid expression", "Error", JOptionPane.ERROR_MESSAGE);
}
}
});
frame.add(minusButton);
JButton multiplyButton = new JButton("*");
multiplyButton.setBounds(220, 200, width, height);
multiplyButton.addActionListener(e -> {
String expression = textField.getText();
if (!expression.isEmpty()) {
textField.setText("");
try {
ScriptEngineManager manager = new ScriptEngineManager();
ScriptEngine engine = manager.getEngineByName("js");
Object result = engine.eval(expression);
textField.setText(result.toString());
} catch (ScriptException ex) {
JOptionPane.showMessageDialog(frame, "Invalid expression", "Error", JOptionPane.ERROR_MESSAGE);
}
}
});
frame.add(multiplyButton);
JButton divideButton = new JButton("/");
divideButton.setBounds(220, 270, width, height);
divideButton.addActionListener(e -> {
String expression = textField.getText();
if (!expression.isEmpty()) {
textField.setText("");
try {
ScriptEngineManager manager = new ScriptEngineManager();
ScriptEngine engine = manager.getEngineByName("js");
Object result = engine.eval(expression);
textField.setText(result.toString());
} catch (ScriptException ex) {
JOptionPane.showMessageDialog(frame, "Invalid expression", "Error", JOptionPane.ERROR_MESSAGE);
}
}
});
frame.add(divideButton);
// 添加等号按钮
JButton equalButton = new JButton("=");
equalButton.setBounds(150, 290, width, height);
equalButton.addActionListener(e -> {
String expression = textField.getText();
if (!expression.isEmpty()) {
try {
ScriptEngineManager manager = new ScriptEngineManager();
ScriptEngine engine = manager.getEngineByName("js");
Object result = engine.eval(expression);
textField.setText(result.toString());
} catch (ScriptException ex) {
JOptionPane.showMessageDialog(frame, "Invalid expression", "Error", JOptionPane.ERROR_MESSAGE);
}
}
});
frame.add(equalButton);
frame.setLayout(null);
frame.setVisible(true);
}
}
```
最后,我们需要显示计算器按钮运算后的结果。我们可以使用Java的Swing库中的JTextField来实现。在每个计算按钮的ActionListener中,我们使用Java的计算表达式库来计算表达式的结果,并将结果显示在JTextField中。
以上就是一个简易的带小数点的计算器的设计和编写过程。希望对你有所帮助!
相关推荐
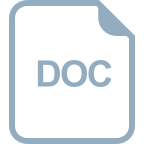
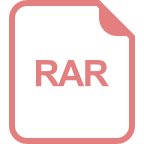















