androidbmi体质指数计算器代码
时间: 2023-09-25 13:06:22 浏览: 189
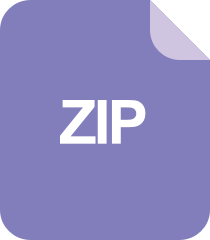
Android代码-BMI计算器
### 回答1:
以下是一个简单的 Android BMI体质指数计算器代码:
MainActivity.java:
```
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
EditText height, weight;
Button calculate;
TextView result;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
height = findViewById(R.id.height);
weight = findViewById(R.id.weight);
calculate = findViewById(R.id.calculate);
result = findViewById(R.id.result);
calculate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
double h = Double.parseDouble(height.getText().toString());
double w = Double.parseDouble(weight.getText().toString());
double bmi = w / (h * h);
String message = "";
if (bmi < 18.5) {
message = "您的BMI指数为" + bmi + ",属于偏瘦";
} else if (bmi >= 18.5 && bmi < 24) {
message = "您的BMI指数为" + bmi + ",属于正常";
} else if (bmi >= 24 && bmi < 28) {
message = "您的BMI指数为" + bmi + ",属于超重";
} else {
message = "您的BMI指数为" + bmi + ",属于肥胖";
}
result.setText(message);
}
});
}
}
```
activity_main.xml:
```
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="身高(米):" />
<EditText
android:id="@+id/height"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="numberDecimal" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="体重(公斤):" />
<EditText
android:id="@+id/weight"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="numberDecimal" />
<Button
android:id="@+id/calculate"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="计算BMI" />
<TextView
android:id="@+id/result"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="16dp" />
</LinearLayout>
```
这个代码使用了一个简单的 UI,包括身高和体重的文本框,一个“计算BMI”按钮以及用于显示结果的文本框。当用户点击“计算BMI”按钮时,程序将计算BMI并显示结果。
### 回答2:
以下是使用Java语言编写的Android BMI(体质指数)计算器代码:
```java
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private EditText editTextWeight;
private EditText editTextHeight;
private Button buttonCalculate;
private TextView textViewResult;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editTextWeight = findViewById(R.id.editTextWeight);
editTextHeight = findViewById(R.id.editTextHeight);
buttonCalculate = findViewById(R.id.buttonCalculate);
textViewResult = findViewById(R.id.textViewResult);
buttonCalculate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
calculateBMI();
}
});
}
private void calculateBMI() {
double weight = Double.parseDouble(editTextWeight.getText().toString());
double height = Double.parseDouble(editTextHeight.getText().toString());
double bmi = weight / (height * height);
String result;
if (bmi < 18.5) {
result = "过轻";
} else if (bmi >= 18.5 && bmi < 25) {
result = "正常";
} else if (bmi >= 25 && bmi < 30) {
result = "过重";
} else {
result = "肥胖";
}
textViewResult.setText("BMI指数:" + bmi + "\n体质指数:" + result);
}
}
```
这段代码是一个简单的BMI计算器应用程序,用户可以输入体重和身高,计算并显示BMI指数和体质指数。BMI的计算方法是体重(千克)除以身高(米)的平方。根据BMI指数的范围,可以确定体质指数的等级,例如过轻、正常、过重和肥胖。当用户点击"计算"按钮时,会调用`calculateBMI()`方法进行计算,并将结果显示在`textViewResult`文本视图中。
(此回答仅提供了基础的计算器实现,并未包含更多的功能和错误处理。如果需要实现更完整的BMI计算器,请根据实际需求进行修改和完善。)
### 回答3:
下面是一个简单的Android BMI体质指数计算器的示例代码:
MainActivity.java:
```java
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
EditText heightInput, weightInput;
Button calculateButton;
TextView resultText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
heightInput = findViewById(R.id.height_input);
weightInput = findViewById(R.id.weight_input);
calculateButton = findViewById(R.id.calculate_button);
resultText = findViewById(R.id.result_text);
calculateButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// 获取身高和体重输入值
float height = Float.parseFloat(heightInput.getText().toString());
float weight = Float.parseFloat(weightInput.getText().toString());
// 计算BMI值
float bmi = weight / (height * height);
// 显示结果
resultText.setText("您的BMI指数为:" + bmi);
}
});
}
}
```
activity_main.xml:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/height_input"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="身高(米)"
android:inputType="numberDecimal"/>
<EditText
android:id="@+id/weight_input"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/height_input"
android:hint="体重(千克)"
android:inputType="numberDecimal"/>
<Button
android:id="@+id/calculate_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/weight_input"
android:text="计算BMI"/>
<TextView
android:id="@+id/result_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/calculate_button"
android:padding="16dp"/>
</RelativeLayout>
```
这个示例代码创建了一个包含输入身高和体重的EditText,一个计算按钮和一个用于显示结果的TextView。当用户点击计算按钮时,会从输入框中获取身高和体重的值,并计算BMI指数。最后,结果将显示在TextView中。请确保在Android Studio中创建一个新的项目,并将这些代码粘贴到相应的文件中。
阅读全文
相关推荐


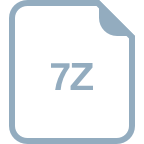
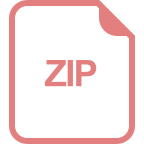
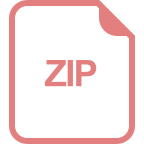
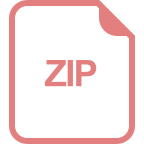
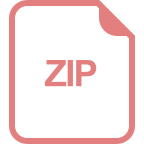
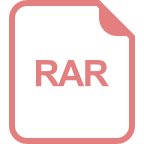
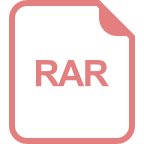