写一个16进制颜色和RGB颜色可以相互转换的程序,并将整个代码用tkinter写出一个界面,界面包含16进制颜色和rgb颜色的输入框,并有一个可以执行转换的按钮,并在转换完成后,在界面上显示对应颜色
时间: 2024-05-11 18:15:42 浏览: 58
好的,这是一个很好的编程问题。以下是一个Python代码的例子,可以实现16进制颜色和RGB颜色之间的转换,并使用tkinter创建一个简单的界面:
```python
import tkinter as tk
def hex_to_rgb(hex_color):
"""Converts a hexadecimal color string to RGB color tuple."""
hex_color = hex_color.lstrip('#')
return tuple(int(hex_color[i:i+2], 16) for i in (0, 2, 4))
def rgb_to_hex(rgb_color):
"""Converts an RGB color tuple to hexadecimal color string."""
return '#{:02x}{:02x}{:02x}'.format(*rgb_color)
def convert_color():
"""Converts the color from one format to the other."""
try:
if hex_color_entry.get():
rgb_color = hex_to_rgb(hex_color_entry.get())
rgb_color_entry.delete(0, tk.END)
rgb_color_entry.insert(0, str(rgb_color))
elif rgb_color_entry.get():
rgb_color = eval(rgb_color_entry.get())
hex_color_entry.delete(0, tk.END)
hex_color_entry.insert(0, rgb_to_hex(rgb_color))
else:
raise ValueError("Please enter either a hexadecimal or RGB color.")
color_label.config(bg=hex_color_entry.get())
except Exception as e:
error_label.config(text=str(e))
# Create the main window
root = tk.Tk()
root.title("Color Converter")
# Create the input fields and labels
hex_color_label = tk.Label(root, text="Hexadecimal Color:")
hex_color_entry = tk.Entry(root)
rgb_color_label = tk.Label(root, text="RGB Color:")
rgb_color_entry = tk.Entry(root)
convert_button = tk.Button(root, text="Convert", command=convert_color)
color_label = tk.Label(root, width=30, height=10, bg="#ffffff")
error_label = tk.Label(root, fg="red")
# Add the widgets to the window
hex_color_label.grid(row=0, column=0, padx=5, pady=5)
hex_color_entry.grid(row=0, column=1, padx=5, pady=5)
rgb_color_label.grid(row=1, column=0, padx=5, pady=5)
rgb_color_entry.grid(row=1, column=1, padx=5, pady=5)
convert_button.grid(row=2, column=0, columnspan=2, padx=5, pady=5)
color_label.grid(row=3, column=0, columnspan=2, padx=5, pady=5)
error_label.grid(row=4, column=0, columnspan=2, padx=5, pady=5)
# Run the main loop
root.mainloop()
```
这个程序创建了一个简单的tkinter窗口,其中包含一个文本框和一个按钮,用于输入和转换颜色。当用户点击“转换”按钮时,程序将检查输入框中的值,并将其转换为另一种格式的颜色。转换完成后,程序将在界面上显示对应的颜色。
请注意,此代码仅用于演示目的。在实际应用中,您应该包括错误检查和更全面的测试。
阅读全文
相关推荐



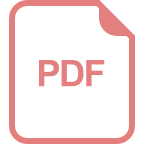
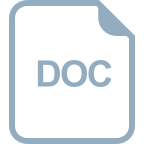








