用Python写一个爬取网页文字的代码
时间: 2024-06-08 20:00:46 浏览: 22
可以使用Python中的requests和BeautifulSoup库来实现网页文字的爬取。以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
text = soup.get_text()
print(text)
```
这个代码首先使用requests库获取网页内容,然后使用BeautifulSoup库将网页内容解析为一个树形结构。接着,我们可以使用get_text()方法获取网页中的所有文本内容。最后,将文本内容打印出来。
需要注意的是,这个示例代码只会获取网页中的所有文本内容,如果你需要获取指定的某些文本,可以通过BeautifulSoup提供的各种选择器来实现。
相关问题
请用python代码写一个爬取网页文字信息的代码
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com" # 需要爬取的网页链接
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
text = soup.get_text() # 获取网页中的文字信息
print(text)
帮我写一个爬取网页文字的python程序
好的,以下是一个简单的爬取网页文字的Python程序,使用requests和BeautifulSoup库:
```python
import requests
from bs4 import BeautifulSoup
# 目标网页的URL
url = 'http://www.example.com'
# 发送请求,获取网页HTML源代码
response = requests.get(url)
html = response.text
# 使用BeautifulSoup解析HTML源代码,提取出所有文本信息
soup = BeautifulSoup(html, 'html.parser')
text = soup.get_text()
# 输出提取出的文本信息
print(text)
```
在上面的程序中,我们首先指定了目标网页的URL,并使用requests库向该网页发送请求,获取网页的HTML源代码。然后,使用BeautifulSoup库解析HTML源代码,提取出其中的文本信息,并将提取出的文本信息输出到控制台。
相关推荐
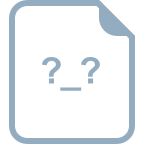
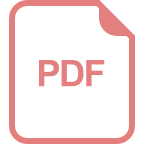












