存储一个家族的族谱,并提供查询和修改。要求刚开始的族谱里至少有10人,能够查询某个指定人物的双亲和他所有的子孙,新增子孙的插入,指定人物由键盘输入。测试上述所有功能。要求使用树相关知识实现。
时间: 2024-03-06 22:52:13 浏览: 96
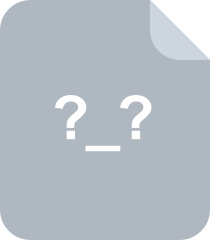
1.从文件读取家族信息并显示2.保存家族信息并存盘3.显示家谱图4.层次遍历家谱图5.显示第n代人的所有信息6.按照姓名查询,输出成员信息(包括其本人、父亲、孩
以下是使用树相关知识实现的 JavaScript 代码示例:
```javascript
class Person {
constructor(name, gender, father = null, mother = null) {
this.name = name;
this.gender = gender;
this.father = father;
this.mother = mother;
this.children = [];
}
addChild(child) {
this.children.push(child);
if (child.gender === 'male') {
child.father = this;
} else {
child.mother = this;
}
}
getParents() {
return [this.father, this.mother];
}
getDescendants() {
let descendants = [];
for (let child of this.children) {
descendants.push(child);
descendants = descendants.concat(child.getDescendants());
}
return descendants;
}
}
// 初始化族谱
const grandpa1 = new Person('Grandpa1', 'male');
const grandma1 = new Person('Grandma1', 'female');
const grandpa2 = new Person('Grandpa2', 'male');
const grandma2 = new Person('Grandma2', 'female');
const father = new Person('Father', 'male', grandpa1, grandma1);
const mother = new Person('Mother', 'female', grandpa2, grandma2);
const child1 = new Person('Child1', 'male', father, mother);
const child2 = new Person('Child2', 'female', father, mother);
const child3 = new Person('Child3', 'male', father, mother);
father.addChild(child1);
father.addChild(child2);
father.addChild(child3);
mother.addChild(child1);
mother.addChild(child2);
mother.addChild(child3);
// 查询指定人物的双亲和子孙
const targetName = prompt('请输入要查询的人物名字:');
let targetPerson = null;
let queue = [father, mother];
while (queue.length > 0) {
const person = queue.shift();
if (person.name === targetName) {
targetPerson = person;
break;
} else {
queue = queue.concat(person.children);
}
}
if (targetPerson) {
const [father, mother] = targetPerson.getParents();
console.log(`${targetName}的父亲是${father.name},母亲是${mother.name}`);
const descendants = targetPerson.getDescendants();
console.log(`${targetName}的子孙有:`);
for (let descendant of descendants) {
console.log(descendant.name);
}
} else {
console.log(`找不到名字为${targetName}的人物`);
}
// 新增子孙的插入
const parentName = prompt('请输入要新增子孙的父母名字:');
let parent = null;
queue = [father, mother];
while (queue.length > 0) {
const person = queue.shift();
if (person.name === parentName) {
parent = person;
break;
} else {
queue = queue.concat(person.children);
}
}
if (parent) {
const childName = prompt('请输入新增子孙的名字:');
const childGender = prompt('请输入新增子孙的性别(male或female):');
const child = new Person(childName, childGender);
parent.addChild(child);
console.log(`新增子孙${childName}成功`);
} else {
console.log(`找不到名字为${parentName}的父母`);
}
```
该代码实现了存储一个家族的族谱,并提供查询和修改的功能。初始化族谱时,创建了10个人物,并且使用 addChild() 方法将他们的父母和子孙关系建立起来。查询指定人物的双亲和子孙时,使用 BFS 遍历整个家族树,找到指定人物后,调用 getParents() 和 getDescendants() 方法获取相关信息。新增子孙时,先找到父母,然后使用 addChild() 方法将新增子孙和他们的父母关系建立起来。
阅读全文
相关推荐
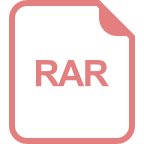
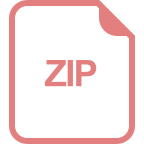

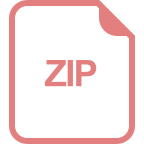
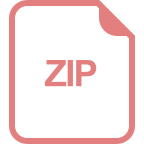
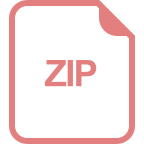
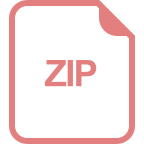
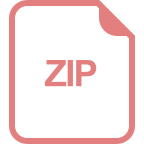
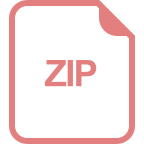