用java语言写一个茶叶销售系统
时间: 2023-12-09 20:05:57 浏览: 78
好的,以下是一个简单的使用Java语言编写的茶叶销售系统代码示例,实现了添加产品,展示产品列表,购买产品等基本功能:
```java
import java.util.ArrayList;
import java.util.Scanner;
class Product {
String name;
double price;
int stock;
Product(String name, double price, int stock) {
this.name = name;
this.price = price;
this.stock = stock;
}
}
class TeaSalesSystem {
ArrayList<Product> products;
TeaSalesSystem() {
products = new ArrayList<>();
}
void addProduct(String name, double price, int stock) {
products.add(new Product(name, price, stock));
}
void showProducts() {
System.out.println("Product list:");
for (int i = 0; i < products.size(); i++) {
Product product = products.get(i);
System.out.println((i+1) + ". " + product.name + " - $" + product.price + " - " + product.stock + " in stock");
}
}
void buyProduct(int index, int quantity) {
Product product = products.get(index-1);
if (product.stock < quantity) {
System.out.println("Sorry, only " + product.stock + " left in stock");
return;
}
double totalPrice = product.price * quantity;
System.out.println("You have purchased " + quantity + " " + product.name + " for $" + totalPrice);
product.stock -= quantity;
}
}
public class Main {
public static void main(String[] args) {
TeaSalesSystem system = new TeaSalesSystem();
system.addProduct("Green tea", 5.99, 10);
system.addProduct("Black tea", 4.99, 15);
system.addProduct("Oolong tea", 6.99, 8);
system.showProducts();
Scanner input = new Scanner(System.in);
System.out.print("Enter product index to purchase: ");
int index = input.nextInt();
System.out.print("Enter quantity to purchase: ");
int quantity = input.nextInt();
system.buyProduct(index, quantity);
system.showProducts();
}
}
```
此代码创建了一个`Product`类来表示茶叶产品,以及一个`TeaSalesSystem`类来管理产品列表和购买操作。在`main()`方法中创建了一个系统实例,并添加了三种茶叶产品。`showProducts()`方法用于列出产品列表,`buyProduct()`方法用于购买产品并更新库存。使用`Scanner`类获取用户输入来选择要购买的产品和数量。
阅读全文
相关推荐
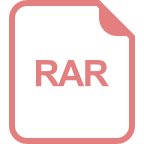
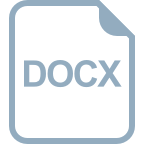
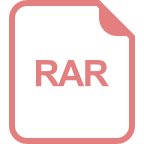
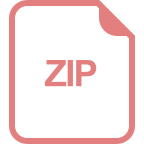
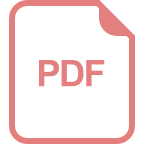
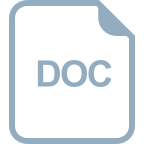
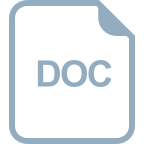
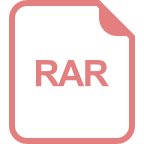
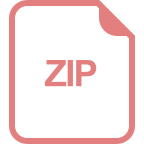
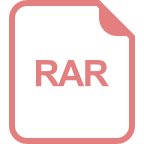
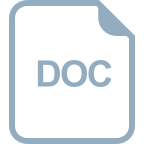
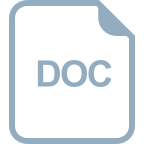
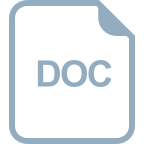
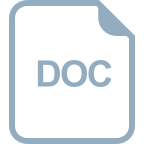
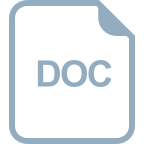
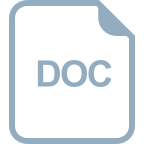
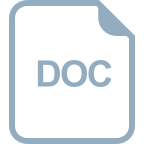