div距离页面底部触发事件
时间: 2023-07-01 13:25:23 浏览: 211
你可以使用 `window.innerHeight` 和 `document.documentElement.scrollTop` 来计算出当前窗口的可见高度和页面滚动的距离,然后再加上元素本身的高度和距离页面顶部的距离,就可以判断元素是否滚动到了页面底部。示例代码如下:
```javascript
var element = document.getElementById('yourElement');
window.addEventListener('scroll', function() {
var elementDistanceToTop = element.getBoundingClientRect().top;
var windowHeight = window.innerHeight;
var scrollDistance = document.documentElement.scrollTop;
if (elementDistanceToTop - windowHeight <= 0) {
// 元素已经滚动到了页面底部
console.log('到达底部');
}
});
```
其中`'yourElement'`是你要监听的元素的ID。当元素滚动到页面底部时,控制台会输出`'到达底部'`。
相关问题
div距离页面底部只触发一次事件
要让div距离页面底部只触发一次事件,可以使用一个变量来记录是否已经触发过事件,然后在事件处理函数中进行判断。例如:
```javascript
let isTriggered = false;
window.addEventListener('scroll', function() {
const div = document.querySelector('div');
const rect = div.getBoundingClientRect();
const windowHeight = window.innerHeight;
const distanceToBottom = windowHeight - rect.bottom;
if (distanceToBottom >= 0 && !isTriggered) {
// 触发事件
console.log('div到达页面底部');
isTriggered = true;
}
});
```
在这段代码中,我们定义了一个`isTriggered`变量来记录是否已经触发过事件,默认为`false`。在滚动事件处理函数中,我们通过`getBoundingClientRect`方法获取div元素的位置信息,计算出它距离页面底部的距离`distanceToBottom`。如果`distanceToBottom`小于等于0,说明div已经到达页面底部,此时我们判断`isTriggered`是否为`false`,如果是,表示还没有触发过事件,就执行相应的操作,并将`isTriggered`设置为`true`,表示已经触发过事件。这样,当div再次到达页面底部时,由于`isTriggered`已经为`true`,事件处理函数就不会再执行了,从而实现了只触发一次事件的效果。
vue 如何判断iframe内的页面是否滚动到底部
### 回答1:
可以通过以下方式判断iframe内的页面是否滚动到底部:
1. 获取iframe元素
```javascript
const iframe = document.getElementById('my-iframe')
```
2. 获取iframe内部的document对象
```javascript
const iframeDoc = iframe.contentDocument || iframe.contentWindow.document
```
3. 判断iframe内部的页面是否滚动到底部
```javascript
const isIframeScrollToBottom = iframeDoc.documentElement.scrollHeight - iframeDoc.documentElement.scrollTop === iframeDoc.documentElement.clientHeight
```
如果isIframeScrollToBottom为true,则表示iframe内部的页面已经滚动到底部。
### 回答2:
在Vue中,我们可以通过以下步骤来判断iframe内的页面是否滚动到底部:
1. 首先,我们需要获取到iframe元素,可以通过`document.getElementById`来获取iframe元素的引用。
2. 接下来,我们可以通过`contentWindow`属性来获取iframe内部窗口的引用,以便后续操作。
3. 通过`contentWindow.document.documentElement.scrollHeight`和`contentWindow.document.documentElement.clientHeight`属性,可以得到iframe内部文档的总高度和显示窗口的高度。
4. 判断滚动高度是否等于(或大于)文档总高度减去显示窗口的高度,即`contentWindow.document.documentElement.scrollTop >= (contentWindow.document.documentElement.scrollHeight - contentWindow.document.documentElement.clientHeight)`。
5. 如果上述条件成立,则表示iframe内的页面已经滚动到底部。
以下是一个示例的Vue方法,用于判断iframe内的页面是否滚动到底部:
```javascript
methods: {
checkIframeScroll() {
const iframe = document.getElementById('myIframe');
const contentWindow = iframe.contentWindow;
const scrollHeight = contentWindow.document.documentElement.scrollHeight;
const clientHeight = contentWindow.document.documentElement.clientHeight;
const scrollTop = contentWindow.document.documentElement.scrollTop;
if (scrollTop >= (scrollHeight - clientHeight)) {
console.log('滚动到底部');
} else {
console.log('未滚动到底部');
}
}
}
```
请注意,以上示例中的`myIframe`是根据iframe元素的id属性来获取引用的,您需要根据实际情况进行调整。另外,示例中的判断方法是在某个Vue方法中调用的,您可以根据需求来触发该方法。
### 回答3:
在Vue中判断iframe内的页面是否滚动到底部,可以通过以下步骤实现:
1. 在Vue组件中,使用ref属性给iframe元素添加一个唯一的引用名称,例如ref="myIframe"。
2. 在mounted()生命周期钩子函数中获取该iframe元素,并添加滚动事件监听器。
3. 在滚动事件监听器中判断iframe内页面的滚动位置是否已经到达底部。
具体代码如下:
```javascript
<template>
<div>
<iframe ref="myIframe" src="https://example.com"></iframe>
</div>
</template>
<script>
export default {
mounted() {
// 获取iframe元素并添加滚动事件监听器
const iframe = this.$refs.myIframe;
iframe.addEventListener("scroll", this.checkScrollBottom);
},
methods: {
checkScrollBottom() {
// 判断iframe内页面是否到达底部
const iframe = this.$refs.myIframe;
const scrollTop = iframe.scrollTop; // 滚动距离
const scrollHeight = iframe.scrollHeight; // 内容高度
const clientHeight = iframe.clientHeight; // 可视区域高度
if (scrollTop + clientHeight >= scrollHeight) {
console.log("已滚动到底部");
// 执行滚动到底部后的操作
}
},
},
};
</script>
```
注意:如果iframe内页面和外部页面不在同一个域,可能会受到同源策略的限制,无法直接获取内部页面的滚动位置。在此情况下,可以使用postMessage方法进行跨域通信,让内部页面向外部页面发送滚动位置信息,然后在外部页面中进行判断。
阅读全文
相关推荐
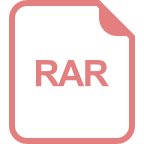
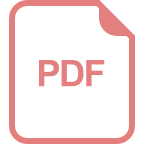
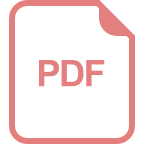
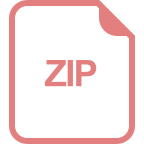
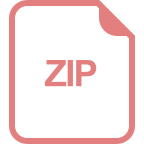
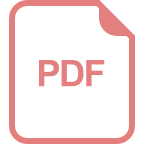
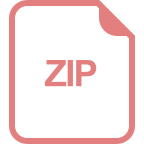
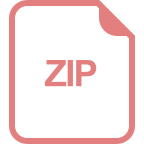
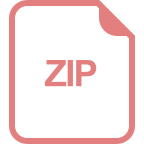
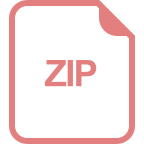
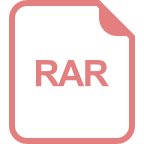
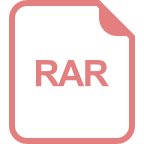
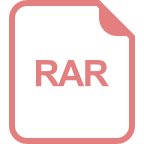
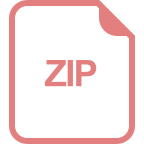
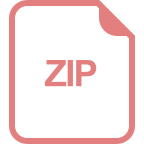
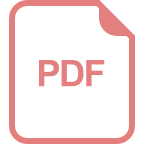
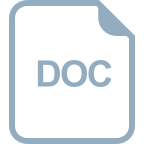