完成函数sortInsert的编写,该函数将nd指向的一个Node结点按num从小到大的顺序插入到head指向的单向链表中,并返回新链表。 样例输入:2 6 4 13 7 9 5 12 样例输出:2 4 5 6 7 9 12 13 C++
时间: 2023-12-30 08:04:58 浏览: 162
下面是一份可能的实现,你可以参考一下:
```cpp
struct Node {
int num;
Node* next;
};
Node* sortInsert(Node* head, Node* nd) {
if (head == nullptr || nd == nullptr) {
return nullptr;
}
if (nd->num < head->num) {
nd->next = head;
return nd;
}
Node* ptr = head;
while (ptr->next != nullptr && ptr->next->num < nd->num) {
ptr = ptr->next;
}
nd->next = ptr->next;
ptr->next = nd;
return head;
}
int main() {
Node* head = nullptr;
// 读入链表节点
int num;
while (cin >> num) {
Node* nd = new Node();
nd->num = num;
nd->next = nullptr;
head = sortInsert(head, nd);
}
// 输出链表
Node* ptr = head;
while (ptr != nullptr) {
cout << ptr->num << ' ';
ptr = ptr->next;
}
// 释放链表节点
ptr = head;
while (ptr != nullptr) {
Node* next = ptr->next;
delete ptr;
ptr = next;
}
return 0;
}
```
该实现中,sortInsert函数的核心思路是遍历链表,找到nd应该插入的位置,然后将nd插入到相应的位置。特别地,如果nd应该插在链表头部,直接将nd作为新的头节点返回即可。
如果你不熟悉链表的操作,可以先看一下链表的基本概念和操作,然后再来阅读本实现。
阅读全文
相关推荐
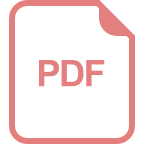
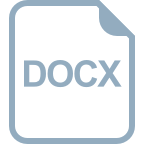
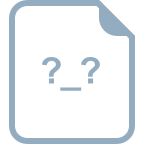
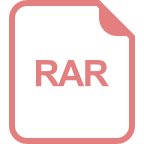
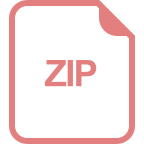
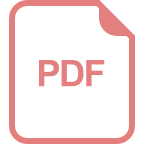
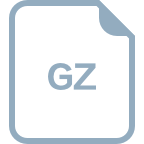
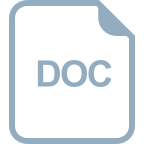
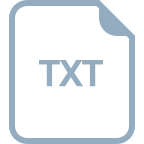
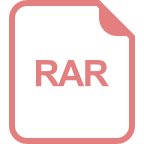
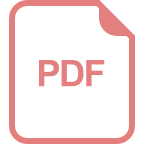
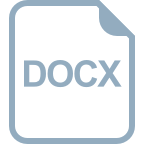
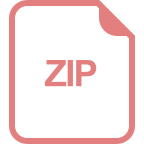
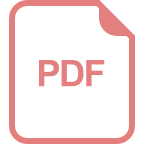
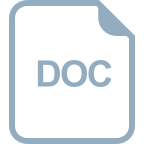
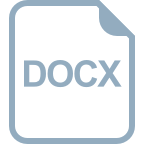