第八次上机: 利用std::thread、std::mutex、std::binary_semaphore完成读写问题的C++代码。模拟实现多个读线程可同时读操作临界区(每次至多5个)、一个写线程写操作临界区。
时间: 2024-01-22 13:02:42 浏览: 222
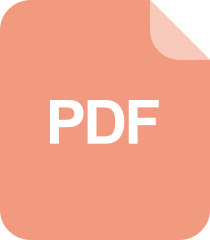
C++11 std::shared_ptr总结与使用示例代码详解
很高兴为你解答这个问题!这是一个关于使用std::thread、std::mutex、std::binary_semaphore实现读写问题的C代码的问题。对于多个读线程可以同时进行读操作临界区的模拟实现,我们可以使用一个写线程进行写操作临界区。为了实现这个过程,我们可以利用互斥量进行线程同步和std::binary_semaphore进行信号量控制。具体实现方法可以参见以下代码:
```
#include <iostream>
#include <thread>
#include <mutex>
#include <chrono>
#include <semaphore.h>
using namespace std;
//定义读写临界区的共享变量
int shared_variable = 0;
//定义互斥量和信号量
mutex mtx;
binary_semaphore semaphore(5);
//定义读线程函数
void read_function(int id){
while(true){
semaphore.acquire(); //信号量操作,控制读线程数量
mtx.lock(); //加锁进入临界区
cout << "Read thread " << id << " reads: " << shared_variable << endl;
this_thread::sleep_for(chrono::milliseconds(2000)); //休眠2秒钟,模拟读的过程
mtx.unlock(); //离开临界区
semaphore.release(); //信号量操作,释放读线程数量
}
}
//定义写线程函数
void write_function(){
while(true){
mtx.lock(); //加锁进入临界区
shared_variable++; //对临界区进行写操作
cout << "Write thread writes: " << shared_variable << endl;
this_thread::sleep_for(chrono::milliseconds(3000)); //休眠3秒钟,模拟写的过程
mtx.unlock(); //离开临界区
}
}
int main(){
//创建五个读线程和一个写线程
thread read_thread_1(read_function, 1);
thread read_thread_2(read_function, 2);
thread read_thread_3(read_function, 3);
thread read_thread_4(read_function, 4);
thread read_thread_5(read_function, 5);
thread write_thread(write_function);
//等待所有线程执行完毕
read_thread_1.join();
read_thread_2.join();
read_thread_3.join();
read_thread_4.join();
read_thread_5.join();
write_thread.join();
return 0;
}
```
希望我的回答能够对你有所帮助!
阅读全文
相关推荐
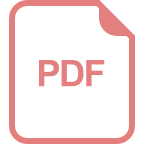
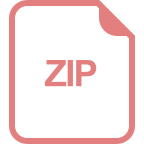















