如果把char bufForReadWrite[] = "ContentToWrite"; size_t bufSize = strlen(bufForReadWrite);//测量出读写缓冲区大小改成char *bufForReadWrite = (char *)malloc(1024 * 1024 * 100);怎么计算bufSize
时间: 2024-02-25 17:59:35 浏览: 18
如果将读写缓冲区的大小改为动态分配的 `char *bufForReadWrite = (char *)malloc(1024 * 1024 * 100);`,那么可以使用 `strlen()` 函数来计算字符串的长度是不准确的,因为它只适用于以 null 字符结尾的字符串。而这里动态分配的缓冲区并不是以 null 字符结尾的字符串。
可以使用 `strlen()` 函数来计算字符串的长度,但是需要在写入缓冲区之前记录一下缓冲区的大小,例如:
```
char *bufForReadWrite = (char *)malloc(1024 * 1024 * 100);
size_t bufSize = 1024 * 1024 * 100;
```
这样就可以在后续的代码中使用 `bufSize` 来表示缓冲区的大小,而不必依赖于 `strlen()` 函数。注意,如果使用了动态分配的缓冲区,需要在使用完后使用 `free()` 函数来释放内存,避免内存泄漏。例如:
```
free(bufForReadWrite);
```
这会释放之前动态分配的缓冲区,以便其他程序可以使用这部分内存。
相关问题
这样算计算读写速度吗?#include <stdio.h> #include <string.h> #include <sys/stat.h> #include <fcntl.h> #include <unistd.h> #include <sys/time.h> int main(int argc, char *argv[]) { char filename[] = "aFile"; // 即将创建和打开的文件名 char *bufForReadWrite = (char *)malloc(1024 * 1024 * 100); // 申请100MB空间作为读写缓冲区 size_t bufSize = sizeof(bufForReadWrite); //测量出读写缓冲区大小 int fd; //用于存放open函数返回的文件描述符 struct timeval tv_start, tv_end; // 用于存放开始和结束时间 // 打开或创建文件并写入数据 fd = open(filename, O_RDWR | O_CREAT, 0644); // 创建并打开文件,可读可写,创建权限为644 if(fd < 0) { // 创建文件失败时返回 perror("Unable to create file"); return -1; } gettimeofday(&tv_start, NULL); // 记录写入开始时间 ssize_t sizeWritten = write(fd, (void *)bufForReadWrite, bufSize); printf("Write: %ld bytes to %s\n", sizeWritten, filename); close(fd); // 写入完毕后关闭文件 // 打开文件并读取数据 fd = open(filename, O_RDWR); // 打开文件后的权限为可读可写 if(fd < 0) { //打开文件失败时返回 perror("Unable to open file"); return -1; } ssize_t sizeRead = read(fd, (void *)bufForReadWrite, bufSize); gettimeofday(&tv_end, NULL); // 记录读取结束时间 printf("Read: %ld bytes from %s, read content:%s\n", sizeRead, filename, bufForReadWrite); close(fd); // 读完毕后关闭文件 // 计算读写速度 double time = (tv_end.tv_sec - tv_start.tv_sec) * 1000.0; // 将时间转为豪秒 time += (tv_end.tv_usec - tv_start.tv_usec) / 1000.0; // 加上微秒部分的时间 double speed__write_read = (sizeWritten / (1024.0 * 1024.0)) / (time / 1000.0); // 读写速度 printf("Read speed: %.2f MB/s\n", speed_write_read); return 0; }
这段代码是用来测试磁盘读写速度的。它首先创建一个文件并写入数据,然后再读取该文件中的数据,并计算出读写速度。具体来说,代码中使用了系统调用函数open、write和read来进行文件操作,使用了结构体timeval来记录时间,使用了malloc函数动态申请内存空间。需要注意的是,代码中的变量名speed__write_read应该改为speed_write_read。
void getCmdResult(const char* cmd, char** resultbuf, size_t* bufsize) { FILE* fp = popen(cmd, "r"); if (fp) { *resultbuf = malloc(*bufsize); fgets(*resultbuf, *bufsize, fp); pclose(fp); } } HUAYI_BOOL TcpObtainServervalue(int count, ...) { char* resultbuf = NULL; size_t bufsize = 1024; char cmd[TEMP_STR_LEN]; int counter = 0; va_list args; va_start(args, count); strcpy(cmd, "cat /tmp/returnValue"); do { if (counter > 10) return HUAYI_FALSE; else if (counter) sleep(1); if (resultbuf) free(resultbuf); getCmdResult(cmd, &resultbuf, &bufsize); counter++; } while (!strlen(resultbuf)); char* token = strtok(resultbuf, " "); int i = 0; while (token != NULL && i < count) { char* str = va_arg(args, char*); strcpy(str, token); token = strtok(NULL, " "); i++; } va_end(args); unlink("/tmp/returnValue"); free(resultbuf); return HUAYI_TRUE; } char name1[128]; char name2[128]; char name3[128]; TcpObtainServervalue(3,name1,name2,name3); printf("%s,%s,%s",name1,name2,name3);strtok分割不确定是空格分割还是换行分割
在你的代码中,`strtok` 函数使用空格作为分隔符来分割字符串。所以在这种情况下,`strtok` 会将 `resultbuf` 字符串按照空格进行分割。
然而,你可能需要注意的是,`fgets` 函数读取的内容会包含换行符。所以如果 `resultbuf` 字符串以换行符结尾,那么 `strtok` 在分割时会将换行符也作为分隔符,导致分割结果不符合预期。
如果你希望在 `strtok` 中同时支持空格和换行符作为分割符,你可以修改代码如下:
```c
char* token = strtok(resultbuf, " \n"); // 使用空格和换行符作为分隔符
```
这样,`strtok` 就会按照空格和换行符进行分割。
相关推荐
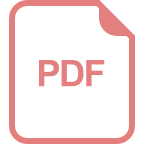
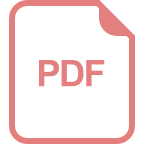
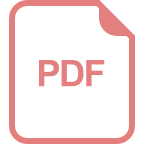












