c#chart控件怎么刷新曲线图
时间: 2023-09-19 19:13:28 浏览: 281
要刷新C#的Chart控件上的曲线图,可以使用Chart控件的Invalidate或者Refresh方法。这两个方法都可以强制Chart控件刷新自己,如果控件中的数据已经发生变化,那么就会重新绘制曲线图。
以下是一个示例代码:
```csharp
// 假设chart控件名为chart1
// 这里使用了一个自定义的UpdateChart方法,用于更新曲线图的数据
// 每次更新完数据后,都调用了chart1的Invalidate方法来刷新曲线图
private void UpdateChart()
{
// 更新曲线图的数据
// ...
// 刷新曲线图
chart1.Invalidate();
}
```
另外,需要注意的是,如果Chart控件中的数据量比较大,或者曲线图的绘制比较复杂,那么频繁地调用Invalidate方法可能会影响性能。此时,可以考虑在更新数据后,只刷新需要更新的部分,而不是整个Chart控件。具体实现方式可以参考Chart控件的Region属性和Invalidate方法的重载版本。
相关问题
chart控件怎么清除曲线图
要清除C#的Chart控件上的曲线图,可以使用Chart控件的Series.Clear方法,这个方法可以清空Chart控件中所有的Series对象,也就是清除所有的曲线图。
以下是一个示例代码:
```csharp
// 假设chart控件名为chart1
// 这里使用了一个自定义的ClearChart方法,用于清除曲线图
// 调用chart1的Series.Clear方法清除所有的曲线图
private void ClearChart()
{
// 清除所有的曲线图
chart1.Series.Clear();
}
```
另外,需要注意的是,如果只想清除某个Series对象对应的曲线图,而不是清除所有的曲线图,可以使用Series.Points.Clear方法,这个方法可以清空Series对象中所有的数据点,也就是清除对应的曲线图。
以下是一个示例代码:
```csharp
// 假设chart控件名为chart1,需要清除的Series对象名为series1
// 调用series1的Points.Clear方法清除该Series对象对应的曲线图
private void ClearSeries()
{
// 清除series1对应的曲线图
chart1.Series["series1"].Points.Clear();
}
```
需要注意的是,如果Series对象中还有其他的属性,比如颜色、线型等,这些属性不会被清除。如果需要清除这些属性,可以在调用Series.Clear或者Series.Points.Clear方法前,手动将这些属性设置为默认值。
c# chart绘制曲线图 demo
以下是一个基本的 C# Chart 绘制曲线图的示例代码:
```csharp
using System.Windows.Forms.DataVisualization.Charting;
// 创建一个 Chart 控件
Chart chart1 = new Chart();
// 设置 Chart 控件的属性
chart1.Size = new System.Drawing.Size(500, 300);
chart1.ChartAreas.Add("MainChartArea");
chart1.Series.Add("Series1");
chart1.Series["Series1"].ChartType = SeriesChartType.Spline;
chart1.Series["Series1"].BorderWidth = 3;
// 添加数据点
chart1.Series["Series1"].Points.AddXY(1, 10);
chart1.Series["Series1"].Points.AddXY(2, 20);
chart1.Series["Series1"].Points.AddXY(3, 15);
chart1.Series["Series1"].Points.AddXY(4, 25);
// 将 Chart 控件添加到窗体上
this.Controls.Add(chart1);
```
以上代码创建了一个大小为 500x300 的 Chart 控件,在其中添加了一个名为 "Series1" 的曲线图系列,并添加了四个数据点。你可以根据需要对 Chart 控件进行更多的自定义和配置。
相关推荐
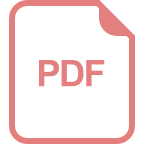












