在jupyterlab中'/home/gqb/Jupyter'这个目录下创建文件夹,在保存后,通过钩子函数,可以获取到文件路径,可以把文件内容读出来,再把它写到特定的目录下,比如'/home/gqb/Jupyter/server/Book/'这个目录,创建jupyter-book工程,并且配置文件自动生成。
时间: 2023-12-31 18:03:23 浏览: 69
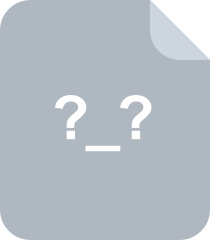
Jupyter文件存储到想要文件夹,而不是默认安装文件夹
好的,下面是一个在JupyterLab中实现你要求的示例代码:
```python
import os
import shutil
import subprocess
# JupyterLab保存目录
jupyterlab_dir = '/home/gqb/Jupyter'
# 目标目录
target_dir = '/home/gqb/Jupyter/server/Book'
# 工程配置文件路径
project_config_file = '/home/gqb/Jupyter/server/Book/_config.yml'
def create_directory(directory):
# 创建目录
os.makedirs(directory, exist_ok=True)
def copy_file(src, dest):
# 复制文件
shutil.copy2(src, dest)
def write_to_project_config(file_path):
# 获取文件名
file_name = os.path.basename(file_path)
# 写入工程配置文件
with open(project_config_file, 'a') as f:
f.write(file_name + '\n')
def post_save_hook(file_path):
# 读取文件内容
with open(file_path, 'r') as f:
file_content = f.read()
# 获取文件名
file_name = os.path.basename(file_path)
# 将文件内容写入目标目录
target_file_path = os.path.join(target_dir, file_name)
with open(target_file_path, 'w') as f:
f.write(file_content)
# 写入工程配置文件
write_to_project_config(target_file_path)
# 创建Jupyter Book工程
subprocess.run(['jupyter-book', 'create', target_dir])
# 示例:在保存文件时调用钩子函数
saved_file_path = '/home/gqb/Jupyter/my_notebook.ipynb'
post_save_hook(saved_file_path)
```
在上述示例中,你需要做以下操作:
1. 将 `jupyterlab_dir` 替换为你的JupyterLab保存目录。
2. 将 `target_dir` 替换为你想要将文件内容复制到的目标目录。
3. 将 `project_config_file` 替换为你想要生成的工程配置文件路径。
然后,在保存文件时调用 `post_save_hook()` 函数,并将保存的文件路径作为参数传递给它。这将触发钩子函数,读取文件内容,将文件内容写入目标目录,并将目标文件的路径写入工程配置文件中。最后,该示例还会使用 `subprocess` 模块调用 `jupyter-book` 命令来创建Jupyter Book工程。
请确保你已经在系统中正确安装了Jupyter Book,并且可以在命令行中运行 `jupyter-book` 命令。
阅读全文
相关推荐
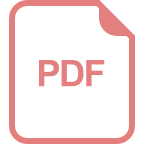
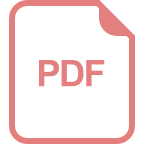
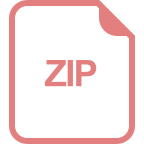
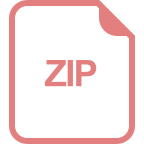
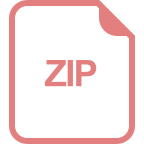
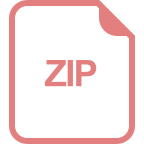
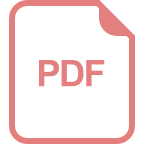
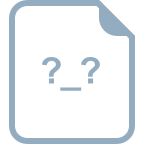
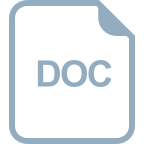
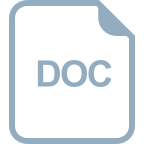
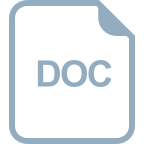
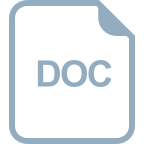
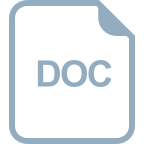

