vue中如何实现在某一区域中多个元素随机定位显示且不重叠给出示例并判断不能重叠
时间: 2024-02-20 17:01:58 浏览: 255
在Vue中实现在某一区域中多个元素随机定位显示且不重叠的方法有很多,以下是一种可能的实现方式:
1. 在Vue中定义一个数组,数组中包含多个元素,每个元素代表一个需要显示的元素,包括其大小、位置、颜色等属性。
2. 在Vue组件的`mounted()`生命周期函数中,使用JavaScript获取需要显示元素的容器的宽高,以及每个元素的宽高。
3. 使用JavaScript生成随机位置,并判断该位置是否与已有元素重叠。如果重叠,则重新生成位置,直到不重叠为止。
4. 将每个元素的位置信息存储到Vue数据中,使用`v-for`指令渲染每个元素并设置其样式,实现多个元素随机定位显示且不重叠的效果。
以下是一个简单示例代码:
```html
<template>
<div class="container">
<div v-for="(item, index) in items" :key="index" :style="getStyle(item)">
{{ item.text }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
items: [
{ text: 'A', width: 50, height: 50 },
{ text: 'B', width: 50, height: 50 },
{ text: 'C', width: 50, height: 50 },
{ text: 'D', width: 50, height: 50 },
{ text: 'E', width: 50, height: 50 }
],
containerWidth: 0,
containerHeight: 0
};
},
mounted() {
// 获取容器宽高
const container = this.$el.querySelector('.container');
this.containerWidth = container.offsetWidth;
this.containerHeight = container.offsetHeight;
// 随机生成位置
this.items.forEach(item => {
item.left = this.random(0, this.containerWidth - item.width);
item.top = this.random(0, this.containerHeight - item.height);
});
// 遍历每个元素,确保不重叠
for (let i = 0; i < this.items.length; i++) {
for (let j = i + 1; j < this.items.length; j++) {
const item1 = this.items[i];
const item2 = this.items[j];
// 判断是否重叠
if (this.isOverlap(item1, item2)) {
// 重新生成位置
item2.left = this.random(0, this.containerWidth - item2.width);
item2.top = this.random(0, this.containerHeight - item2.height);
// 重新遍历前面的元素,确保不重叠
j = i;
}
}
}
},
methods: {
getStyle(item) {
return {
width: `${item.width}px`,
height: `${item.height}px`,
left: `${item.left}px`,
top: `${item.top}px`,
background: item.color || '#ccc'
};
},
random(min, max) {
return Math.floor(Math.random() * (max - min + 1) + min);
},
isOverlap(item1, item2) {
if (
item1.left + item1.width > item2.left &&
item1.left < item2.left + item2.width &&
item1.top + item1.height > item2.top &&
item1.top < item2.top + item2.height
) {
return true;
}
return false;
}
}
};
</script>
```
在上面的示例中,`items`数组包含五个元素,每个元素表示一个方块,包括`text`、`width`、`height`、`left`、`top`等属性。在`mounted()`生命周期函数中,首先获取容器的宽高,然后遍历每个元素,随机生成位置,并遍历前面的元素,确保不重叠。最后使用`v-for`指令渲染每个元素,并根据元素的位置信息设置其样式,实现多个元素随机定位显示且不重叠的效果。
阅读全文
相关推荐
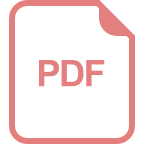
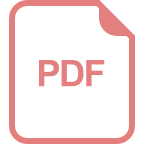
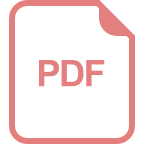













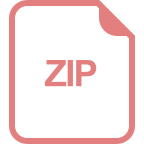
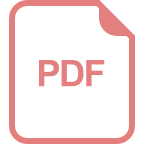
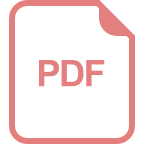