生成CSV文件
时间: 2023-08-19 11:17:02 浏览: 90
好的,生成 CSV 文件的代码已经包含在上面的示例代码中了,这里再给出一些详细的说明。生成 CSV 文件的过程可以分为以下几个步骤:
1. 打开文件:使用 Python 的内置函数 open() 打开一个文件,可以指定文件名、打开模式和编码方式等参数,比如:
```python
with open('weather.csv', 'w', newline='', encoding='utf-8-sig') as f:
# ...
```
在上述代码中,文件名为 'weather.csv',打开模式为写入模式('w'),newline='' 表示写入时不需要额外的换行符,编码方式为 utf-8-sig。
2. 创建 CSV writer:使用 csv 库创建一个 writer 对象,可以指定文件对象和其他参数,比如:
```python
writer = csv.writer(f)
```
在上述代码中,我们将文件对象 f 作为参数传递给 csv.writer() 函数,然后将返回的 writer 对象保存在变量 writer 中。
3. 写入标题行:使用 writer 对象的 writerow() 方法写入标题行,比如:
```python
writer.writerow(['日期', '城市', '天气', '最高温度', '最低温度'])
```
在上述代码中,我们将包含标题行的列表作为参数传递给 writerow() 方法,然后将标题行写入到 CSV 文件中。
4. 写入数据行:使用循环遍历数据,依次将每行数据写入 CSV 文件,比如:
```python
for item in data:
date = item['time']
city = item['station']['city']
weather = item['weather']
temp_max = item['temperature']['max']
temp_min = item['temperature']['min']
writer.writerow([date, city, weather, temp_max, temp_min])
```
在上述代码中,我们依次从每个数据项中取出日期、城市、天气、最高温度和最低温度等信息,然后将这些信息组成一个列表,并将该列表作为参数传递给 writerow() 方法,依次写入到 CSV 文件中。
5. 关闭文件:使用 Python 的内置函数 close() 关闭文件,比如:
```python
f.close()
```
在上述代码中,我们使用 close() 方法关闭文件对象 f,释放资源。
综合以上步骤,可以编写如下的代码来生成 CSV 文件:
```python
import csv
filename = 'weather.csv'
with open(filename, 'w', newline='', encoding='utf-8-sig') as f:
writer = csv.writer(f)
writer.writerow(['日期', '城市', '天气', '最高温度', '最低温度'])
for item in data:
date = item['time']
city = item['station']['city']
weather = item['weather']
temp_max = item['temperature']['max']
temp_min = item['temperature']['min']
writer.writerow([date, city, weather, temp_max, temp_min])
```
在以上代码中,我们将要写入的文件名设为 'weather.csv',使用 open() 函数创建文件对象 f,并指定打开模式为写入模式('w'),编码方式为 utf-8-sig。然后,我们使用 csv.writer() 函数创建一个 writer 对象,并将文件对象 f 作为参数传递进去。接着,我们使用 writerow() 方法写入标题行,然后使用循环遍历数据,依次将每行数据写入 CSV 文件。最后,我们使用 close() 方法关闭文件对象 f。
阅读全文
相关推荐
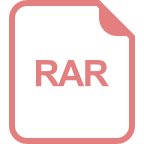
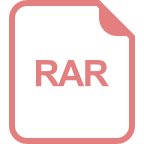
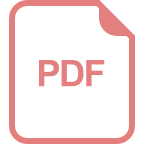
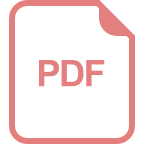
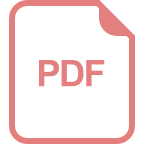
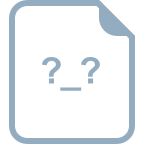
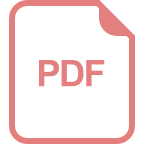
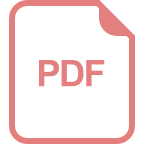
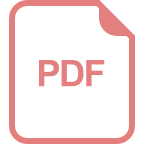
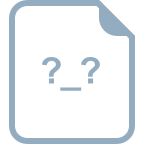
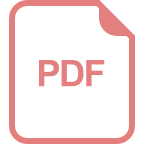
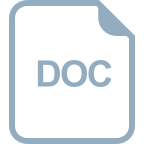