请提供使用vue实现的多级联动菜单的详细示例
时间: 2024-12-25 22:36:47 浏览: 4
在Vue中实现多级联动菜单通常涉及到组件化的设计和嵌套数据结构的理解。下面是一个简单的示例,展示了如何使用Vuex来管理菜单状态,并通过父子组件间的通信触发菜单展开:
首先,我们创建一个基础的菜单项组件(MenuItem.vue):
```html
<template>
<li v-if="item.children">
<a :href="item.url" @click.prevent="$emit('toggleChildren', item)">
{{ item.name }}
<i class="fas fa-caret-down" :class="{ 'fa-caret-right': !expanded }"></i>
</a>
<ul v-show="expanded" ref="childList">
<MenuItem v-for="(child, index) in item.children" :key="index" :item="child" />
</ul>
</li>
</template>
<script>
export default {
props: ['item'],
data() {
return { expanded: false };
},
methods: {
toggleChildren(item) {
this.expanded = !this.expanded;
this.$emit('toggleChild', item);
}
},
};
</script>
```
然后,在主菜单组件(MainMenu.vue)中,我们使用Vuex存储菜单状态:
```html
<template>
<div>
<ul>
<MenuItem v-for="(menu, index) in menus" :key="index" :item="menu" @toggleChild="handleToggleChild" />
</ul>
</div>
</template>
<script>
import { mapState, mapActions } from 'vuex';
import MenuItem from './MenuItem.vue';
export default {
components: { MenuItem },
computed: {
...mapState(['menus']),
},
methods: {
...mapActions(['toggleMenuChild']),
handleToggleChild(item) {
this.toggleMenuChild(item);
}
},
};
</script>
// Vuex store 中的 state 和 action 示例
const state = {
menus: [
// 多级菜单结构
],
};
const mutations = {
setExpanded(state, payload) {
// 更新菜单状态
},
};
const actions = {
toggleMenuChild({ commit }, item) {
commit('setExpanded', { id: item.id, value: !item.expanded });
},
};
</script>
```
在这个例子中,当点击菜单项时,`toggleChild`事件会被发送到父组件,然后通过Vuex中的action触发状态更改,进而控制所有依赖于该状态的视图更新。
阅读全文
相关推荐
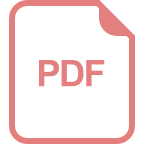
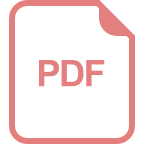
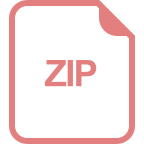

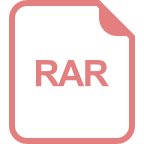
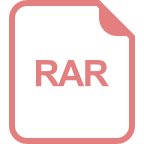
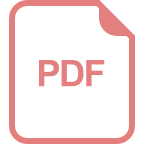
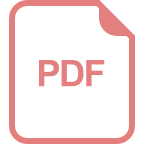
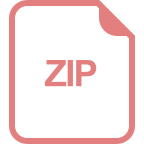
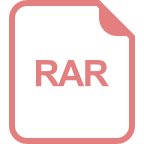
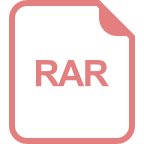
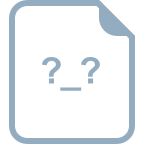
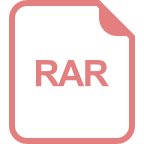
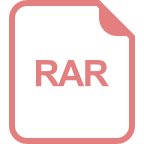
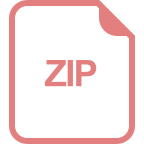
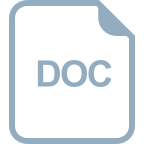
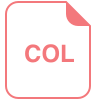
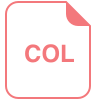

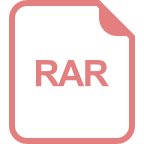