13. 将数据(如"Apple")加密后进行输出。加密规则如下:将单词中的每个字母变成字母表中其后的第五个字母,用流程图写出来
时间: 2024-12-05 09:16:07 浏览: 12
要使用Python编写一个简单的加密程序,我们可以按照以下步骤创建一个流程图:
1. 获取用户输入的数据(在这里是单词 "Apple")。
2. 对每个字符执行加密操作,即将其转换为字母表中其后的第5个字母。
3. 将加密后的字符串输出。
以下是Python代码实现这一过程:
```python
def encrypt_char(char):
# 判断字符是否为大写字母或小写字母
if char.isalpha():
# 如果是英文字母,根据ASCII码计算新的位置并转换
if char.islower():
new_char = chr(((ord(char) - ord('a') + 5) % 26) + ord('a'))
else:
new_char = chr(((ord(char) - ord('A') + 5) % 26) + ord('A'))
else:
# 非字母字符保持不变
new_char = char
return new_char
def caesar_cipher_encrypt(text):
encrypted_text = ""
for char in text:
encrypted_text += encrypt_char(char)
return encrypted_text
# 示例:加密 "Apple"
input_word = "Apple"
encrypted_word = caesar_cipher_encrypt(input_word)
print("Encrypted word:", encrypted_word)
```
现在,让我们用流程图表示这个过程:
```
start
|
V
Input: Apple (User Input)
|
V
For each character:
|
V
Is it a letter?
|
V yes
|
V Encrypt with Caesar Cipher (Shift by 5 positions)
|
V Add to encrypted_text
|
V no (Non-alphabetic characters remain unchanged)
|
V end of loop
|
V
Output: Encrypted_word (display on screen)
|
V
end
```
阅读全文
相关推荐
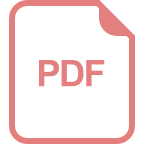
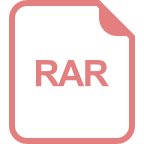
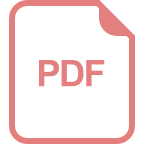
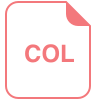
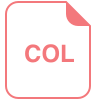
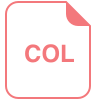
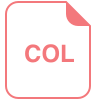
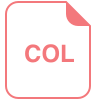
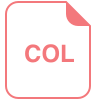
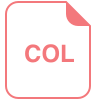
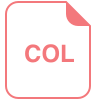
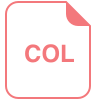
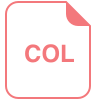
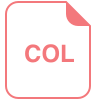
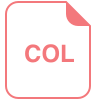
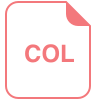
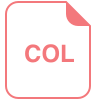
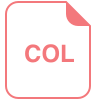
