用JAVA写一个,创建6个学生对象到集合,并按要求遍历输出,学生属性(姓名,年龄,语文,数学,英语),按从高到低输出控制台,总分一样按语文排,语文一样按数学排,数学一样按英语排,英语一样按年龄排,年龄一样按姓名排,如果都一样,认为是同一个学生,不存
时间: 2024-10-09 08:09:08 浏览: 80
首先,我们需要创建一个`Student`类,包含姓名、年龄和三个分数属性。然后,我们将学生对象存储在一个`ArrayList`或`PriorityQueue`(按照特定规则排序)中。下面是使用`PriorityQueue`实现的例子:
```java
import java.util.*;
class Student implements Comparable<Student> {
String name;
int age;
double mathScore;
double englishScore;
double chineseScore;
// 构造函数...
@Override
public int compareTo(Student other) {
int totalScoreComparison = Double.compare(this.totalScore(), other.totalScore());
if (totalScoreComparison != 0) return totalScoreComparison;
int chineseComparison = Double.compare(this.chineseScore, other.chineseScore);
if (chineseComparison != 0) return chineseComparison;
int mathComparison = Double.compare(this.mathScore, other.mathScore);
if (mathComparison != 0) return mathComparison;
int englishComparison = Double.compare(this.englishScore, other.englishScore);
if (englishComparison != 0) return englishComparison;
int ageComparison = Integer.compare(this.age, other.age);
if (ageComparison != 0) return ageComparison;
// 如果所有都一样,则认为是同一个人,这里可以简单地返回0或者抛出异常
return 0; // 返回0表示相等
}
// 计算总分
double totalScore() {
// ...计算公式...
}
// getters and setters...
}
public class Main {
public static void main(String[] args) {
PriorityQueue<Student> students = new PriorityQueue<>();
// 创建并添加6个学生
students.add(new Student("张三", 18, 90.5, 92.0, 88.5));
students.add(new Student("李四", 20, 95.0, 93.0, 90.0));
// ...其他学生...
// 遍历并按要求输出
while (!students.isEmpty()) {
Student currentStudent = students.poll();
System.out.println("姓名:" + currentStudent.name + ", 年龄:" + currentStudent.age +
", 语文:" + currentStudent.chineseScore + ", 数学:" + currentStudent.mathScore +
", 英语:" + currentStudent.englishScore);
}
}
}
```
阅读全文
相关推荐
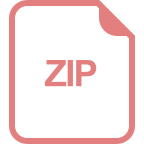
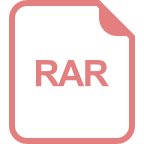
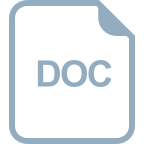












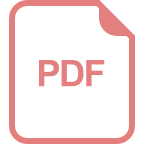
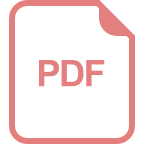
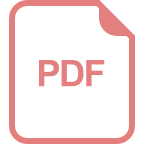
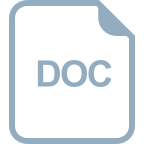