C语言,将数据结构传入函数,函数内对数据结构各个内容修改,请举例
时间: 2024-12-24 21:37:32 浏览: 21
在C语言中,你可以通过将数据结构作为参数传递给函数,让函数能够访问并修改其成员。下面是一个简单的例子,假设我们有一个名为Person的结构体,包含姓名(name)和年龄(age)两个字段:
```c
#include <stdio.h>
// 定义Person结构体
typedef struct {
char name[50];
int age;
} Person;
// 函数,用于修改Person结构体的内容
void updatePerson(Person *person, const char *newName, int newAge) {
// 检查输入是否为空
if (!newName || !newAge) {
printf("Invalid input!\n");
return;
}
// 修改结构体内容
strcpy(person->name, newName);
person->age = newAge;
// 显示更新后的信息
printf("Name updated to: %s\n", person->name);
printf("Age updated to: %d\n", person->age);
}
int main() {
// 创建Person实例
Person john = {"John", 25};
// 将john的地址传递给updatePerson函数
updatePerson(&john, "Jane", 30);
// 主函数结束后,john的改变依然有效
printf("After the function call: Name is %s, Age is %d.\n", john.name, john.age);
return 0;
}
```
在这个例子中,`updatePerson`函数接受一个Person类型的指针,以及新的名字和年龄。它直接操作这个指针所指向的结构体,改变其内容。当函数返回后,主函数main中的john结构体也被相应地更新了。
阅读全文
相关推荐












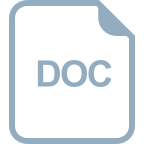





