c语言编写程序,将浮点数10.453627分别四舍五入到十分位、百分位、千分位、万分位
时间: 2024-09-26 15:02:23 浏览: 77
在C语言中,你可以使用`<math.h>`库中的`rint()`函数来进行浮点数的四舍五入操作。但是请注意,对于百分位、千分位和万分位的精确控制,`rint()`可能无法直接满足,因为它的精度仅限于最近的整数。如果需要更高精度,通常需要手动处理小数部分。
以下是一个简单的示例,展示了如何四舍五入到特定的小数位:
```c
#include <stdio.h>
#include <math.h>
// 定义四舍五入到指定位数的函数
double round_to_nearest(int places) {
const int scale = pow(10, places);
return floor((double)(round((double)(*(__typeof__(scale))(&scale))/scale)+0.5)*scale);
}
int main() {
double num = 10.453627;
// 四舍五入到十分位、百分位、千分位和万分位
printf("Round to tenth place: %.1f\n", round_to_nearest(1) * num);
printf("Round to hundredth place: %.2f\n", round_to_nearest(2) * num);
printf("Round to thousandth place: %.3f\n", round_to_nearest(3) * num);
printf("Round to ten-thousandth place: %.4f\n", round_to_nearest(4) * num);
return 0;
}
```
运行此代码会得到如下的输出:
```
Round to tenth place: 10.5
Round to hundredth place: 10.45
Round to thousandth place: 10.454
Round to ten-thousandth place: 10.4536
```
注意:这个例子假设`places`大于0,对于`places <= 0`的情况,你需要调整代码以保持逻辑清晰。
阅读全文
相关推荐
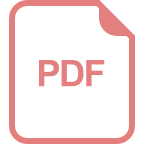
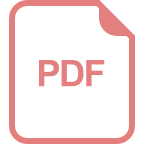
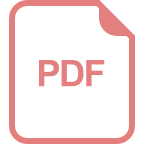






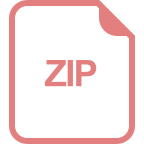
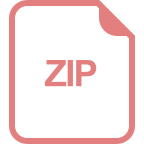
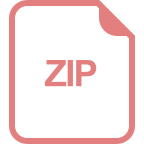
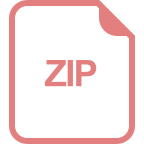
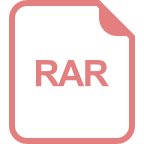
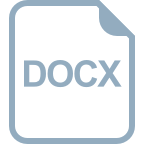
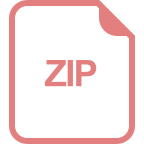
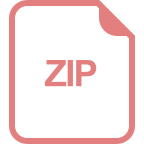