springcloud详细介绍,以及代码示例
时间: 2023-11-05 21:02:28 浏览: 88
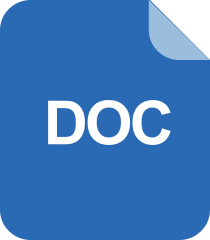
SpringCloud详细介绍

Spring Cloud是基于Spring框架的一套微服务开发工具集。它提供了一系列的工具来帮助开发者快速构建分布式系统,包括配置管理、服务发现、负载均衡、断路器、消息总线、分布式数据存储等功能。
Spring Cloud的核心组件包括:
1. Eureka:服务发现和注册中心,用于管理服务的注册、发现和负载均衡。
2. Ribbon:客户端负载均衡器,用于在客户端上实现负载均衡。
3. Feign:客户端HTTP请求工具,用于简化HTTP请求的编写。
4. Hystrix:断路器,用于实现服务的熔断和降级。
5. Zuul:API网关,用于路由、过滤和负载均衡。
6. Config:配置中心,用于管理应用程序的配置信息。
以下是一个简单的Spring Cloud示例,它演示了如何使用Eureka、Ribbon和Feign构建一个基本的微服务应用程序。
1. 创建一个Spring Boot项目,添加以下依赖:
```
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-ribbon</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
```
2. 在application.properties文件中添加以下配置:
```
spring.application.name=example-service
eureka.client.serviceUrl.defaultZone=http://localhost:8761/eureka
```
3. 创建一个RESTful API接口:
```
@RestController
@RequestMapping("/example")
public class ExampleController {
@Autowired
private ExampleService exampleService;
@GetMapping("/{id}")
public Example getById(@PathVariable Long id) {
return exampleService.getById(id);
}
}
```
4. 创建一个服务接口:
```
@FeignClient(name = "example-service")
public interface ExampleService {
@GetMapping("/example/{id}")
Example getById(@PathVariable Long id);
}
```
5. 在启动类上添加@EnableDiscoveryClient注解,启用服务发现功能:
```
@SpringBootApplication
@EnableDiscoveryClient
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
6. 启动Eureka Server,并将服务注册到Eureka中。
7. 启动多个示例应用程序,它们将自动注册到Eureka中,并使用Ribbon和Feign进行负载均衡和HTTP请求。
阅读全文
相关推荐
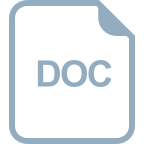

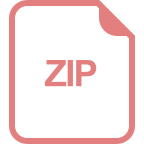
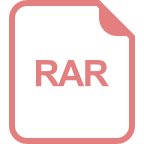
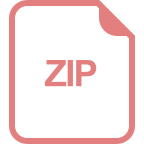
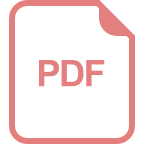
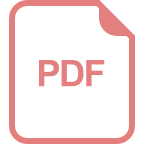
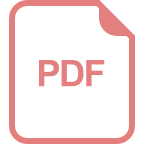
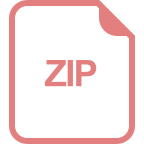
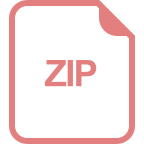
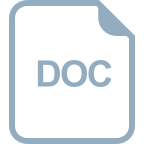
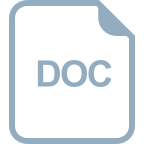
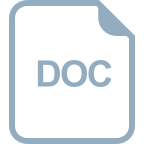

