圣诞树python网页代码
时间: 2024-12-25 21:20:19 浏览: 8
### 使用Python生成圣诞树图形的网页代码
为了实现在网页上显示由Python生成的圣诞树图形,可以采用Flask框架来构建Web应用,并通过HTML/CSS/JavaScript渲染页面。下面是一个完整的解决方案。
#### Flask Web 应用设置
首先安装必要的包:
```bash
pip install flask
```
接着创建`app.py`文件:
```python
from flask import Flask, render_template_string
import random
app = Flask(__name__)
@app.route('/')
def christmas_tree():
tree_html = generate_christmas_tree()
return render_template_string('''
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Christmas Tree</title>
<style>
.tree {
color: green;
font-size: 2rem;
text-align: center;
margin-top: 5%;
}
@keyframes blink {
from { opacity: 1; }
to { opacity: 0;}
}
span.blinking{
animation-name: blink;
animation-duration: 700ms;
animation-timing-function: ease-in-out;
animation-iteration-count: infinite;
animation-direction: alternate;
}
</style>
</head>
<body>
<div class="tree">{{ tree }}</div>
</body>
</html>
''', tree=tree_html)
def generate_christmas_tree():
width = 21
star = '<span style="color:yellow">★</span>'
trunk = '||'
lines = []
for i in range(1, width + 1, 2):
line = '*' * i
if random.choice([True, False]):
pos = (len(line)-1)//2
decorated_line = list(line)
decorated_line[pos] = f'<span class="blinking">{decorated_line[pos]}</span>'
line = ''.join(decorated_line)
padding = ' ' * ((width - len(line)) // 2)
lines.append(padding + line + padding)
# Add the star at top and trunk at bottom.
star_padding = ' ' * ((width - len(star)) // 2)
trunk_padding = ' ' * ((width - len(trunk)) // 2)
lines.insert(0, star_padding + star + star_padding)
lines.append('\n' + trunk_padding + trunk + '\n')
return '<br>'.join(lines)
if __name__ == '__main__':
app.run(debug=True)
```
这段代码定义了一个简单的Flask应用程序,在根路径下返回一个带有动态生成圣诞树图案的HTML文档[^3]。此方法不仅实现了静态图像展示还加入了闪烁效果模拟灯光装饰[^1]。
阅读全文
相关推荐



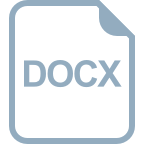
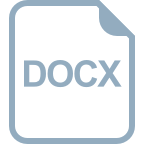
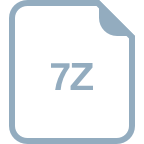
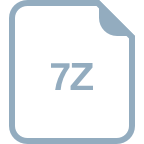
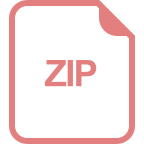
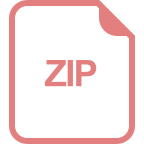
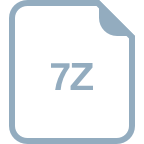