java 导出多个发票格式excel的代码
时间: 2024-03-25 20:36:09 浏览: 68
好的,下面是一个简单的Java代码示例,可以实现导出多个发票格式的Excel文件。
```java
import java.io.FileOutputStream;
import java.util.ArrayList;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class InvoiceExporter {
public static void main(String[] args) {
// 创建需要导出的发票数据
List<Invoice> invoices = createInvoices();
// 导出Excel文件
try (XSSFWorkbook workbook = new XSSFWorkbook()) {
// 创建新的工作表
Sheet sheet = workbook.createSheet("发票列表");
// 创建表头行
Row headerRow = sheet.createRow(0);
Cell cell = headerRow.createCell(0);
cell.setCellValue("发票号码");
cell = headerRow.createCell(1);
cell.setCellValue("客户名称");
cell = headerRow.createCell(2);
cell.setCellValue("发票金额");
// 填充数据行
int rowNum = 1;
for (Invoice invoice : invoices) {
Row row = sheet.createRow(rowNum++);
row.createCell(0).setCellValue(invoice.getNumber());
row.createCell(1).setCellValue(invoice.getCustomer());
row.createCell(2).setCellValue(invoice.getAmount());
}
// 将数据写入Excel文件
FileOutputStream outputStream = new FileOutputStream("invoices.xlsx");
workbook.write(outputStream);
workbook.close();
} catch (Exception e) {
e.printStackTrace();
}
}
// 创建发票数据
private static List<Invoice> createInvoices() {
List<Invoice> invoices = new ArrayList<>();
invoices.add(new Invoice("001", "客户A", 1000));
invoices.add(new Invoice("002", "客户B", 2000));
invoices.add(new Invoice("003", "客户C", 3000));
return invoices;
}
// 发票类
private static class Invoice {
private String number;
private String customer;
private double amount;
public Invoice(String number, String customer, double amount) {
this.number = number;
this.customer = customer;
this.amount = amount;
}
public String getNumber() {
return number;
}
public String getCustomer() {
return customer;
}
public double getAmount() {
return amount;
}
}
}
```
这个示例程序创建了一个包含3个发票的列表,并将列表中的发票信息导出为一个Excel文件。你可以根据需要修改代码,实现更加复杂的发票导出功能。
阅读全文
相关推荐
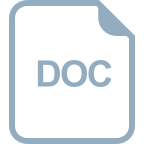
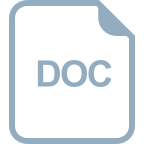
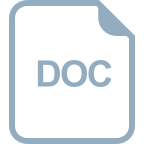
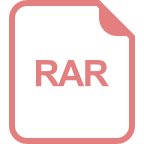
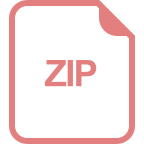
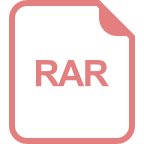
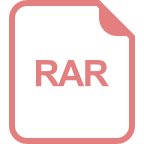
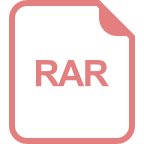
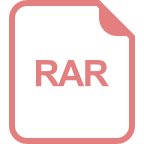
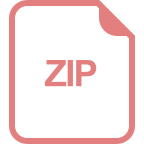
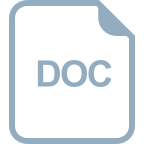
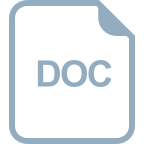
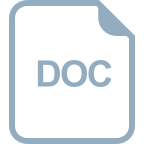
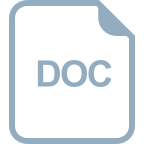
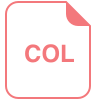
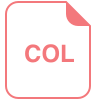
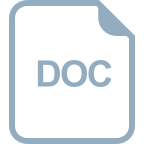
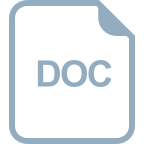