C++中string类型转换为int
时间: 2023-04-06 16:02:42 浏览: 160
可以使用 stoi 函数将 string 类型转换为 int 类型,例如:
```
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "123";
int num = stoi(str);
cout << num << endl;
return 0;
}
```
输出结果为:
```
123
```
相关问题
C++将string类型转化为int类型
可以使用`std::stoi`函数将`string`类型转换为`int`类型。例如:
```c++
#include <iostream>
#include <string>
int main() {
std::string str_num = "123";
int num = std::stoi(str_num);
std::cout << num << std::endl; // 输出 123
return 0;
}
```
需要注意的是,如果`string`类型中包含非数字字符,那么`std::stoi`函数会抛出`std::invalid_argument`异常。如果转换结果超出了`int`类型的取值范围,那么`std::stoi`函数会抛出`std::out_of_range`异常。因此,在使用`std::stoi`函数时,要确保`string`类型中只包含数字字符,并且转换结果不会超出`int`类型的取值范围。
C++中将string类型转换为int, float, double
在C++中,可以使用以下方法将string类型转换为int、float和double类型:
1. 将string转换为int类型:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "123";
int num = std::stoi(str);
std::cout << num << std::endl;
return 0;
}
```
这里使用了std::stoi函数来将string类型转换为int类型。
2. 将string转换为float类型:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
float num = std::stof(str);
std::cout << num << std::endl;
return 0;
}
```
这里使用了std::stof函数来将string类型转换为float类型。
3. 将string转换为double类型:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "3.14159";
double num = std::stod(str);
std::cout << num << std::endl;
return 0;
}
```
这里使用了std::stod函数来将string类型转换为double类型。
以上方法都使用了C++标准库中的stoi、stof和stod函数来实现类型转换。这些函数在解析字符串时会自动处理各种格式错误和异常情况。
阅读全文
相关推荐
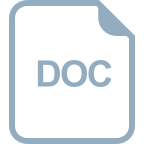
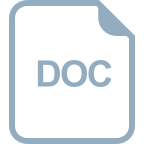
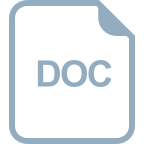
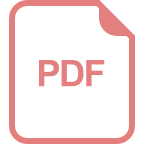












