stack容器实现字符串截取
时间: 2023-10-11 19:13:26 浏览: 60
使用栈容器可以实现字符串截取的功能。具体实现方法如下:
1.定义一个栈容器,用来存储截取后的字符串。
2.遍历原始字符串,当遇到需要截取的字符时,将栈容器中的元素弹出,直到遇到该字符为止。
3.继续遍历原始字符串,将遇到的字符依次压入栈容器中。
4.当遇到下一个需要截取的字符时,重复步骤2和3。
5.最后将栈容器中的元素依次弹出,组成截取后的字符串。
以下是使用栈容器实现字符串截取的示例代码:
```c++
#include <iostream>
#include <string>
#include <stack>
using namespace std;
string subString(string str, char ch) {
stack<char> s;
string result;
for (int i = 0; i < str.length(); i++) {
if (str[i] == ch) {
while (!s.empty()) {
result = s.top() + result;
s.pop();
}
} else {
s.push(str[i]);
}
}
while (!s.empty()) {
result = s.top() + result;
s.pop();
}
return result;
}
int main() {
string str = "Hello,World!";
char ch = ',';
string result = subString(str, ch);
cout << result << endl;
return 0;
}
```
输出结果为:`Hello`
阅读全文
相关推荐
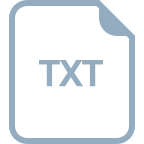
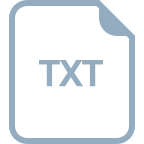
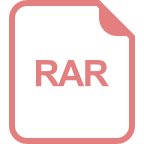
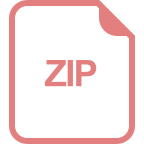
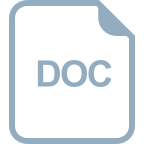
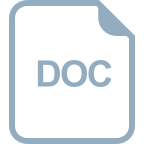
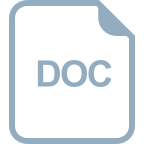
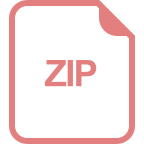