(1)在互联网中查找HTML5+CSS3制作的按钮及源码。 (2)在互联网中查找照片过渡及变换效果的源码。 (3)在上一个实验的源码基础上修改添加制作小组网站Logo图片,添加按钮,打开网页时,将小组成员的图片用变换效果弹出。
时间: 2023-11-28 20:51:14 浏览: 73
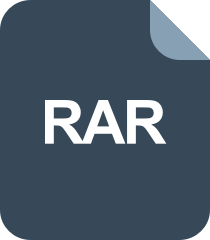
HTML5+CSS3网站设计基础教程_动手实践源代码

(1) 下面是一个简单的 HTML5 + CSS3 制作按钮的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>Button Example</title>
<style>
.button {
display: inline-block;
padding: 10px 20px;
border-radius: 10px;
background-color: #4CAF50;
color: white;
font-size: 18px;
text-decoration: none;
transition: background-color 0.3s ease;
}
.button:hover {
background-color: #3e8e41;
}
</style>
</head>
<body>
<a href="#" class="button">Click me</a>
</body>
</html>
```
(2) 下面是一个图片过渡及变换效果的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>Image Transition Example</title>
<style>
.image-container {
position: relative;
width: 300px;
height: 200px;
overflow: hidden;
}
.image-container img {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: opacity 0.5s ease;
}
.image-container img.active {
opacity: 1;
}
</style>
</head>
<body>
<div class="image-container">
<img src="image1.jpg" class="active">
<img src="image2.jpg">
<img src="image3.jpg">
<img src="image4.jpg">
</div>
<script>
let images = document.querySelectorAll(".image-container img");
let index = 0;
setInterval(() => {
images[index].classList.remove("active");
index = (index + 1) % images.length;
images[index].classList.add("active");
}, 2000);
</script>
</body>
</html>
```
(3) 基于上一个实验的代码,我们可以添加一个小组网站的 Logo 图片和一个弹出小组成员图片的按钮。代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>Image Transition Example</title>
<style>
.image-container {
position: relative;
width: 300px;
height: 200px;
overflow: hidden;
}
.image-container img {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: opacity 0.5s ease;
}
.image-container img.active {
opacity: 1;
}
.logo {
display: block;
margin: 20px auto;
width: 200px;
height: 100px;
background-image: url("logo.png");
background-repeat: no-repeat;
background-size: contain;
}
.button {
display: inline-block;
padding: 10px 20px;
border-radius: 10px;
background-color: #4CAF50;
color: white;
font-size: 18px;
text-decoration: none;
transition: background-color 0.3s ease;
}
.button:hover {
background-color: #3e8e41;
}
.member-image {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 200px;
height: 200px;
border-radius: 50%;
background-repeat: no-repeat;
background-size: cover;
background-position: center;
opacity: 0;
transition: opacity 0.5s ease;
}
.member-image.active {
opacity: 1;
}
</style>
</head>
<body>
<div class="logo"></div>
<div class="image-container">
<img src="image1.jpg" class="active">
<img src="image2.jpg">
<img src="image3.jpg">
<img src="image4.jpg">
</div>
<a href="#" class="button" onclick="showMemberImage()">Show Member Image</a>
<div class="member-image" style="background-image: url('member1.jpg')"></div>
<div class="member-image" style="background-image: url('member2.jpg')"></div>
<div class="member-image" style="background-image: url('member3.jpg')"></div>
<div class="member-image" style="background-image: url('member4.jpg')"></div>
<script>
let images = document.querySelectorAll(".image-container img");
let index = 0;
setInterval(() => {
images[index].classList.remove("active");
index = (index + 1) % images.length;
images[index].classList.add("active");
}, 2000);
function showMemberImage() {
let memberImages = document.querySelectorAll(".member-image");
let index = Math.floor(Math.random() * memberImages.length);
memberImages[index].classList.add("active");
setTimeout(() => {
memberImages[index].classList.remove("active");
}, 5000);
}
</script>
</body>
</html>
```
阅读全文
相关推荐
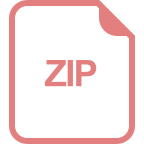
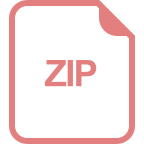
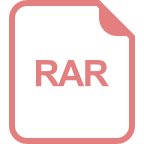
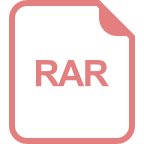
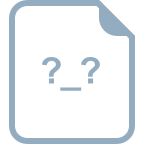
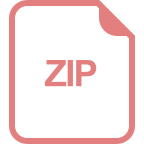
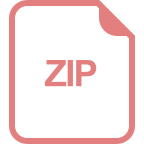
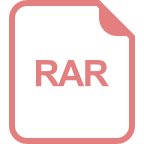
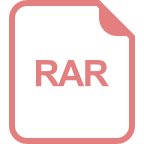
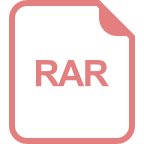
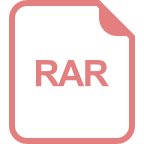
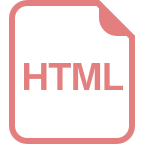
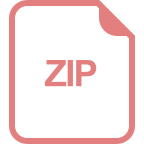