android点击按钮跳转activity
时间: 2023-07-28 12:12:49 浏览: 115
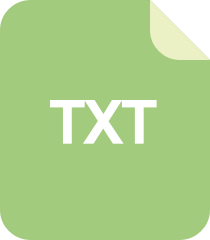
Android 中从activity1跳转到activity2再回到activity所经历的生命周期
在 Android 中,我们可以使用 Intent 类来实现 Activity 之间的跳转。以下是一个简单的示例:
1. 在布局文件中定义一个 Button 组件,并为其添加点击事件:
```
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="跳转到另一个页面"
android:onClick="jumpToAnotherActivity" />
```
2. 在 Java 代码中实现跳转逻辑:
```
public void jumpToAnotherActivity(View view) {
Intent intent = new Intent(this, AnotherActivity.class);
startActivity(intent);
}
```
其中,`AnotherActivity` 是你要跳转到的 Activity 类名,可以替换成你自己的类名。
注意:在 AndroidManifest.xml 文件中,需要为每个 Activity 添加 `<activity>` 标签,以便系统能够识别它们。例如:
```
<activity android:name=".AnotherActivity" />
```
阅读全文
相关推荐
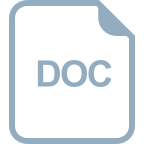
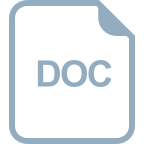
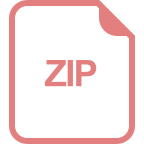














