pandas filter
时间: 2023-05-27 21:01:28 浏览: 141
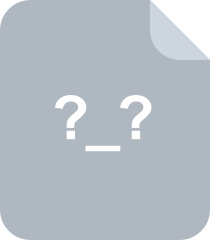
pandas的使用方法
Pandas filter is a method used to select a subset of data from a pandas dataframe based on certain criteria or conditions. It can be used to filter rows or columns based on values in one or multiple columns.
The filter method takes a boolean expression as input and returns a dataframe with only the rows that satisfy the condition. The boolean expression can be created by applying logical operators such as “and”, “or”, and “not” to comparison operators such as “==”, “!=”, “>”, “<”, “>=”, and “<=”.
Syntax of the filter method:
```
df.filter(condition, axis=0)
```
where df is the pandas dataframe, condition is the boolean expression that needs to be evaluated, and axis=0 indicates that the filter needs to be applied to rows.
Example of filtering rows based on a certain condition:
```
import pandas as pd
data = {'Name': ['John', 'Sara', 'Zach', 'Emma', 'David'],
'Age': [25, 28, 21, 30, 35],
'Gender': ['M', 'F', 'M', 'F', 'M'],
'Country': ['USA', 'Australia', 'Canada', 'USA', 'Australia']}
df = pd.DataFrame(data)
# filter rows where age is greater than 25 and country is USA
filtered_df = df[(df['Age'] > 25) & (df['Country'] == 'USA')]
print(filtered_df)
```
Output:
```
Name Age Gender Country
0 John 25 M USA
3 Emma 30 F USA
```
This code filters the rows where age is greater than 25 and country is USA, resulting in a new dataframe with only these two rows.
阅读全文
相关推荐
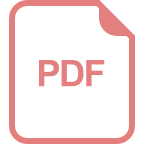
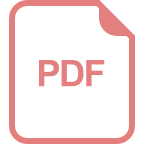





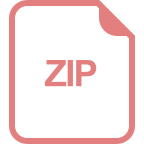
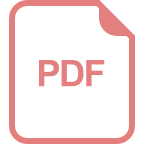









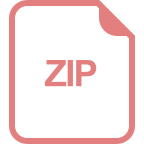