使用c++实现定义一个中文停用词表并输入一个中文句子,删去句子中的停用词
时间: 2024-06-18 07:05:37 浏览: 109
要定义一个中文停用词表并使用 C++ 删除句子中的停用词,需要以下几个步骤:
1. 首先,需要准备好一个中文停用词表,可以将这些词汇存储在一个文件中,例如 stopwords.txt。
2. 然后,在 C++ 中读取这个文件,并将其中的词汇存储在一个字符串数组或者 vector 中。这个数组或者 vector 中的每个元素都是一个停用词。
3. 接下来,输入一个中文句子,可以使用 cin 或者 getline 函数实现。然后,将这个句子分解成单个词汇,可以使用分词库或者手动实现。
4. 最后,遍历分解后的词汇列表,如果某个词汇出现在停用词表中,则将其删除。可以使用 erase 函数从 vector 或者 string 中删除元素。
以下是一个简单的 C++ 代码示例,其中假设停用词表存储在 stopwords.txt 文件中:
```
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
int main() {
// 读取停用词表
ifstream ifs("stopwords.txt");
vector<string> stopwords;
string stopword;
while (ifs >> stopword) {
stopwords.push_back(stopword);
}
// 输入句子
string sentence;
cout << "请输入句子:";
getline(cin, sentence);
// 分解句子并删除停用词
vector<string> words;
string word;
for (int i = 0; i < sentence.size(); i++) {
if (sentence[i] == ' ') {
words.push_back(word);
word = "";
} else {
word += sentence[i];
}
}
words.push_back(word);
for (auto it = words.begin(); it != words.end(); ) {
if (find(stopwords.begin(), stopwords.end(), *it) != stopwords.end()) {
it = words.erase(it);
} else {
it++;
}
}
// 输出结果
cout << "删除停用词后的句子为:";
for (auto word : words) {
cout << word << " ";
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐
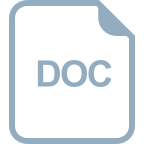
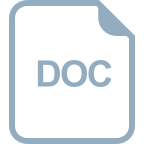
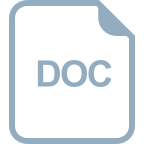
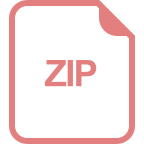
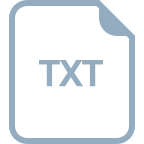
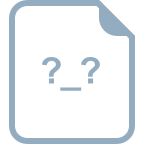
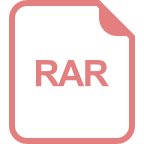
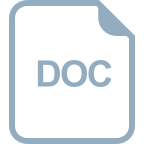
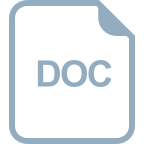
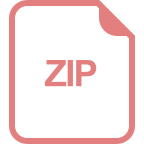
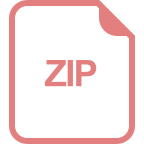
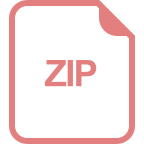
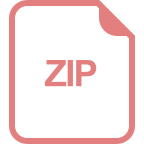
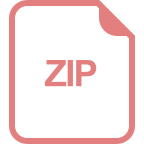