怎样使用Python等编程语言对图像进行处理,提取线条并生成线条图?
时间: 2023-05-26 10:03:21 浏览: 63
使用Python对图像进行处理的常用库有OpenCV、Pillow等,下面以OpenCV为例介绍如何提取线条并生成线条图。
1. 导入OpenCV库
```python
import cv2
```
2. 读取图像
```python
img = cv2.imread('image.jpg')
```
3. 灰度化
```python
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
4. 边缘检测
```python
edges = cv2.Canny(gray, 100, 200)
```
5. 去除噪点
```python
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
edges = cv2.morphologyEx(edges, cv2.MORPH_CLOSE, kernel)
```
6. 提取线条
```python
lines = cv2.HoughLinesP(edges, rho=1, theta=1 * np.pi / 180, threshold=50, minLineLength=50, maxLineGap=30)
```
7. 生成线条图
```python
line_img = np.zeros_like(img)
for line in lines:
x1, y1, x2, y2 = line[0]
cv2.line(line_img, (x1, y1), (x2, y2), (255, 255, 255), 1)
```
完整代码如下:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('image.jpg')
# 灰度化
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 边缘检测
edges = cv2.Canny(gray, 100, 200)
# 去除噪点
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
edges = cv2.morphologyEx(edges, cv2.MORPH_CLOSE, kernel)
# 提取线条
lines = cv2.HoughLinesP(edges, rho=1, theta=1 * np.pi / 180, threshold=50, minLineLength=50, maxLineGap=30)
# 生成线条图
line_img = np.zeros_like(img)
for line in lines:
x1, y1, x2, y2 = line[0]
cv2.line(line_img, (x1, y1), (x2, y2), (255, 255, 255), 1)
# 显示图像
cv2.imshow('Original Image', img)
cv2.imshow('Line Image', line_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
运行代码后会显示原始图像和生成的线条图。
相关推荐
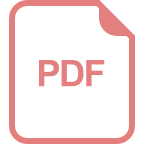
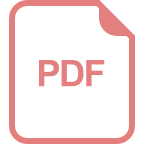
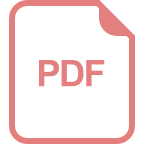
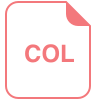













