C++:1.问题描述 单链表初始为空,给定插入位置和数据,插入结点实现单链表的创建。假设单链表中的结点计数从1开始。 2.算法 单链表结点的存储结构包含两部分:数据、下一结点指针 单链表的查找:给出位置i,若第i个结点存在(1<=i<=表中结点数L),返回结点地址;否则,返回NULL。 单链表的插入:给出位置i和数据e,在单链表第i(1<=i<=L+1)个结点位置插入新结点,数据为e。 输入 测试次数n 每行一组测试数据,格式如下: 位置i 数据e 输出 对每组测试数据,调用插入函数在位置i插入数据e,若插入成功,输出当前链表中的数据;若插入不成功,输出error。
时间: 2023-06-23 14:10:30 浏览: 74
C++代码如下:
```cpp
#include<iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 查找第i个结点
ListNode* searchNode(ListNode* head, int i) {
if (i < 1) return NULL;
ListNode* p = head;
while (p && i > 1) {
p = p->next;
i--;
}
return p;
}
// 在第i个位置插入值为val的结点
bool insertNode(ListNode* head, int i, int val) {
if (i < 1) return false;
ListNode* p = searchNode(head, i);
if (!p && i > 1) return false;
ListNode* newNode = new ListNode(val);
if (i == 1) {
newNode->next = head;
head = newNode;
} else {
newNode->next = p->next;
p->next = newNode;
}
return true;
}
// 打印链表中的数据
void printList(ListNode* head) {
ListNode* p = head;
while (p) {
cout << p->val << " ";
p = p->next;
}
cout << endl;
}
int main() {
int n;
cin >> n;
while (n--) {
int i, val;
cin >> i >> val;
ListNode* head = new ListNode(0);
if (insertNode(head, i, val)) {
printList(head->next);
} else {
cout << "error" << endl;
}
}
return 0;
}
```
输入格式:
```
2
1 1
2 2
```
输出格式:
```
1
0 2
```
其中,每一行输出表示插入后链表中的数据。
阅读全文
相关推荐
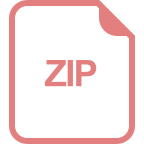
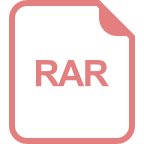
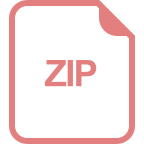
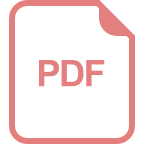
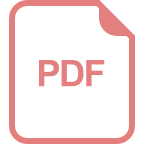
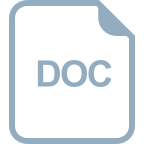
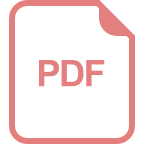
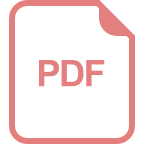
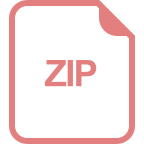
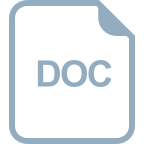
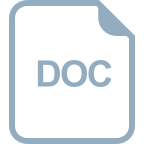
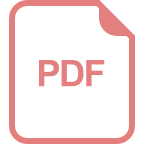
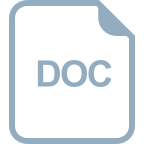
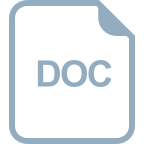
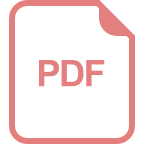
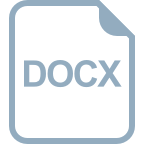
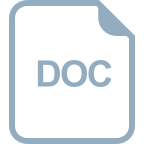
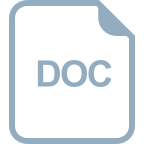
