python Image模块用法
时间: 2023-08-23 09:04:18 浏览: 61
Python中的Image模块是一个用于操作图像的强大工具。它可以处理多种图像格式,包括PNG、JPEG、BMP、GIF等。以下是Image模块的基本用法:
1. 安装Pillow库
在使用Image模块之前,需要先安装Pillow库。可以使用pip命令在终端中安装:
```
pip install Pillow
```
2. 导入Image模块
导入Image模块:
```python
from PIL import Image
```
3. 打开和保存图像
可以使用`Image.open()`方法打开图像文件:
```python
img = Image.open('image.jpg')
```
可以使用`Image.show()`方法显示图像:
```python
img.show()
```
可以使用`Image.save()`方法保存图像:
```python
img.save('new_image.png')
```
4. 调整图像大小
可以使用`Image.resize()`方法调整图像大小:
```python
new_img = img.resize((width, height))
```
其中`width`和`height`为新图像的宽度和高度。
5. 裁剪图像
可以使用`Image.crop()`方法裁剪图像:
```python
box = (left, upper, right, lower)
new_img = img.crop(box)
```
其中`box`是一个元组,表示裁剪矩形的左上角和右下角坐标。
6. 旋转和翻转图像
可以使用`Image.rotate()`方法旋转图像:
```python
new_img = img.rotate(angle)
```
其中`angle`是旋转角度。
可以使用`Image.transpose()`方法翻转图像:
```python
new_img = img.transpose(method)
```
其中`method`可以为`Image.FLIP_LEFT_RIGHT`表示左右翻转,或`Image.FLIP_TOP_BOTTOM`表示上下翻转。
7. 图像滤镜
可以使用`ImageFilter`模块中的滤镜对图像进行处理,例如模糊、边缘检测、锐化等:
```python
from PIL import ImageFilter
new_img = img.filter(ImageFilter.BLUR)
```
其中`ImageFilter.BLUR`表示模糊滤镜。
8. 获取和设置图像属性
可以使用`Image.size`获取图像大小:
```python
width, height = img.size
```
可以使用`Image.format`获取图像格式:
```python
format = img.format
```
可以使用`Image.mode`获取图像模式:
```python
mode = img.mode
```
可以使用`Image.info`获取图像信息:
```python
info = img.info
```
以上是Image模块的基本用法,更多详细信息可以参考Pillow官方文档。
相关推荐
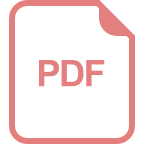
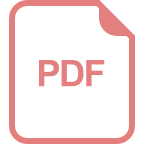
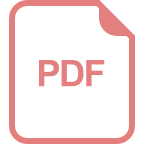














