请用python代码实现链表结构,并用链表实现成对调换,例如:1->2->3->4 转换成 2->1->4->3
时间: 2024-05-08 19:18:02 浏览: 66
好的,以下是Python实现链表的代码:
```python
class Node:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
class LinkedList:
def __init__(self):
self.head = None
def addNode(self, val):
node = Node(val)
if self.head is None:
self.head = node
else:
curr = self.head
while curr.next:
curr = curr.next
curr.next = node
def printList(self):
curr = self.head
while curr:
print(curr.val, end=" ")
curr = curr.next
print()
def swapPairs(self):
if not self.head or not self.head.next:
return self.head
p1, p2 = self.head, self.head.next
self.head = p2
while True:
tmp = p2.next
p2.next = p1
if not tmp or not tmp.next:
p1.next = tmp
break
p1.next = tmp.next
p1, p2 = tmp, tmp.next
return self.head
# test
linked_list = LinkedList()
# add nodes
linked_list.addNode(1)
linked_list.addNode(2)
linked_list.addNode(3)
linked_list.addNode(4)
# print original list
linked_list.printList()
# swap nodes in pairs
linked_list.swapPairs()
# print swapped list
linked_list.printList()
```
输出结果为:
```
1 2 3 4
2 1 4 3
```
请问还有其它问题需要我回答吗?
阅读全文
相关推荐
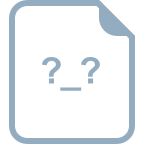
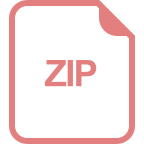
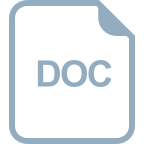
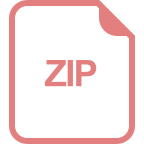
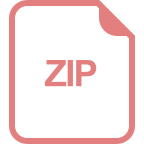
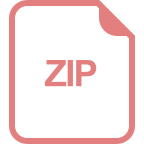
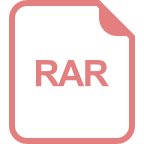
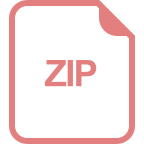
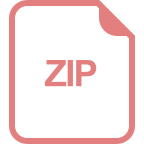
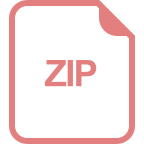
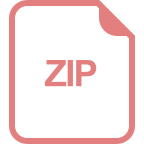
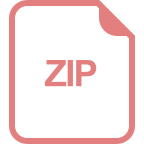
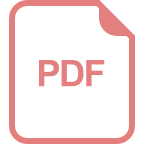
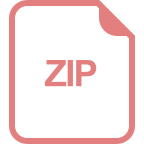