c++正则表达式匹配首元素和尾元素相等的字符串
时间: 2024-10-04 12:04:13 浏览: 29
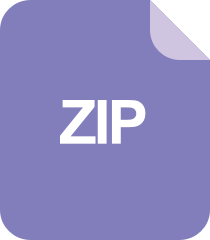
编译原理期末大作业:正则表达式的关系比较(源代码+文档说明)
在C++中,你可以使用标准库`<regex>`来处理正则表达式。如果你想要匹配一个字符串,其中首字符和尾字符相等,可以创建一个简单的正则表达式模式。例如,如果你想检查字符串是否以某个特定字符开始并以该字符结束,你可以这样做:
```cpp
#include <iostream>
#include <regex>
bool matchStartAndEnd(const std::string& str, char expectedChar) {
std::regex pattern("^" + std::string(1, expectedChar) + ".{" + std::to_string(str.length() - 1) + "}" + std::string(1, expectedChar) + "$");
return std::regex_match(str, pattern);
}
int main() {
std::string testString = "apple";
char expected = 'a';
if (matchStartAndEnd(testString, expected)) {
std::cout << "The string starts and ends with " << expected << "." << std::endl;
} else {
std::cout << "The string does not start or end with " << expected << "." << std::endl;
}
return 0;
}
```
在这个例子中,`^` 表示开始,`.` 匹配除换行符以外的任意字符,`{}` 冒号范围表示重复次数,`str.length() - 1` 计算除了首尾字符之外的剩余字符数,最后加上 `$` 表示字符串的结尾。
阅读全文
相关推荐
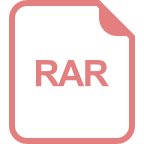
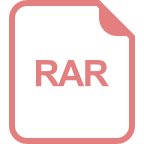

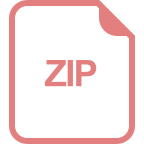
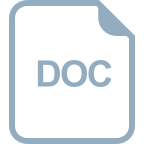
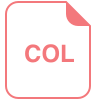
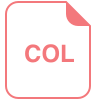
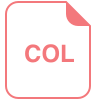
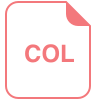
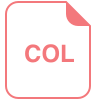
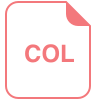
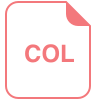
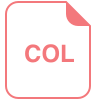
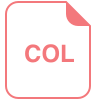
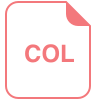


