android实现卡片展开动效实例
时间: 2023-10-25 11:08:52 浏览: 167
以下是一个简单的 Android 卡片展开动效实例:
1. 首先,创建一个布局文件 card_layout.xml,用于显示卡片内容:
```
<LinearLayout
android:id="@+id/cardLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/card_bg"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/titleTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Card Title"
android:textSize="18sp" />
<TextView
android:id="@+id/contentTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Card Content"
android:textSize="14sp" />
</LinearLayout>
```
2. 创建一个展开动画的布局文件 expand_layout.xml,用于显示展开后的卡片内容:
```
<LinearLayout
android:id="@+id/expandLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/card_bg"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/titleTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Card Title"
android:textSize="18sp" />
<TextView
android:id="@+id/contentTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Card Content"
android:textSize="14sp" />
<TextView
android:id="@+id/extraTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Extra Content"
android:textSize="14sp" />
</LinearLayout>
```
3. 创建一个动画类 CardExpandAnimation.java,用于实现卡片的展开和收起:
```
public class CardExpandAnimation extends Animation {
private View view;
private int startHeight;
private int endHeight;
public CardExpandAnimation(View view) {
this.view = view;
this.startHeight = view.getHeight();
this.endHeight = view.getResources().getDimensionPixelSize(R.dimen.card_expand_height);
}
@Override
protected void applyTransformation(float interpolatedTime, Transformation t) {
int newHeight = (int) (startHeight + (endHeight - startHeight) * interpolatedTime);
view.getLayoutParams().height = newHeight;
view.requestLayout();
}
@Override
public boolean willChangeBounds() {
return true;
}
}
```
4. 在 Activity 中,实现卡片的展开和收起:
```
public class MainActivity extends AppCompatActivity {
private LinearLayout cardLayout;
private LinearLayout expandLayout;
private boolean isExpanded = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
cardLayout = findViewById(R.id.cardLayout);
expandLayout = findViewById(R.id.expandLayout);
cardLayout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (isExpanded) {
collapseCard();
} else {
expandCard();
}
}
});
}
private void expandCard() {
CardExpandAnimation animation = new CardExpandAnimation(cardLayout);
animation.setDuration(300);
cardLayout.startAnimation(animation);
expandLayout.setVisibility(View.VISIBLE);
isExpanded = true;
}
private void collapseCard() {
CardExpandAnimation animation = new CardExpandAnimation(cardLayout);
animation.setDuration(300);
cardLayout.startAnimation(animation);
expandLayout.setVisibility(View.GONE);
isExpanded = false;
}
}
```
在这个例子中,我们通过 CardExpandAnimation 类实现了卡片的展开和收起,并在 Activity 中实现了点击事件来触发展开和收起动画。展开后的卡片内容使用了一个单独的布局文件 expand_layout.xml 来实现。
阅读全文
相关推荐
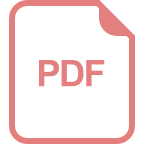
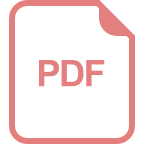
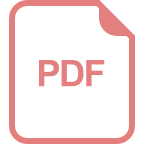
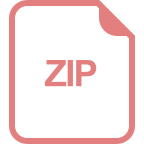
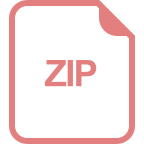
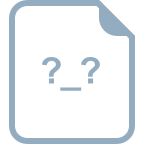
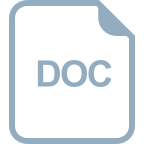
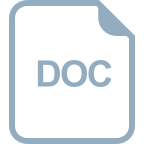
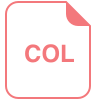
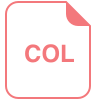
