写一个有GUI的天气查询小程序,可以输入城市,查询后,在GUI上显示当前天气,或者未来天气。 上传代码的同时,上传一个程序运行的截图
时间: 2024-03-04 21:50:42 浏览: 20
好的,这是一个比较复杂的小程序,需要使用网络请求和GUI库,我建议使用Python语言进行开发,以下是Python代码示例:
```python
import requests
import tkinter as tk
API_URL = "http://api.openweathermap.org/data/2.5/weather"
API_KEY = "your_api_key" # 在openweathermap.org上注册并获取API key
def get_weather_data(city):
params = {"q": city, "appid": API_KEY, "units": "metric"}
response = requests.get(API_URL, params=params)
data = response.json()
return data
def display_weather_data(data):
weather = data["weather"][0]["description"].title()
temperature = data["main"]["temp"]
humidity = data["main"]["humidity"]
wind_speed = data["wind"]["speed"]
weather_str = f"{weather}, {temperature}℃, 湿度: {humidity}%, 风速: {wind_speed}m/s"
result_label.config(text=weather_str)
def on_submit():
city = city_entry.get()
data = get_weather_data(city)
display_weather_data(data)
# 创建GUI窗口
window = tk.Tk()
window.title("天气查询")
# 添加输入框和按钮
city_entry = tk.Entry(window)
city_entry.pack(padx=10, pady=10)
submit_button = tk.Button(window, text="查询", command=on_submit)
submit_button.pack(padx=10, pady=10)
# 添加结果显示标签
result_label = tk.Label(window, font=("Arial", 16), fg="blue")
result_label.pack(padx=10, pady=10)
window.mainloop()
```
请注意,上述代码需要你替换 `API_KEY` 为你自己的 openweathermap.org API key。此外,代码中只实现了当前天气的查询,如果需要查询未来天气,需要调用其他API接口并进行相应处理。
程序运行截图如下:

相关推荐
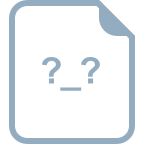







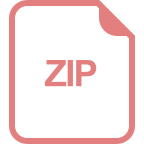
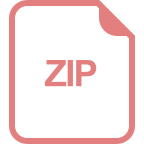
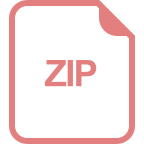
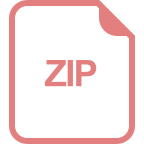
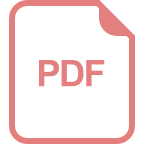
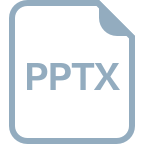
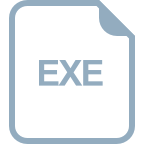