springboot 引入缓存机制
时间: 2023-10-14 14:14:53 浏览: 44
在Spring Boot中使用缓存可以显著提高应用程序的性能和响应速度。Spring Boot提供了对多种缓存技术的支持,包括EhCache、Redis、Caffeine等。下面是一个示例,演示如何在Spring Boot中使用EhCache。
1. 添加依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
```
2. 配置缓存:
在application.properties文件中添加以下配置:
```properties
# 开启缓存
spring.cache.type=ehcache
```
3. 创建缓存:
在你的服务类上添加@Cacheable注解,并指定缓存名称,如:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserRepository userRepository;
@Cacheable(value = "users")
@Override
public User getUserById(Long id) {
Optional<User> optionalUser = userRepository.findById(id);
return optionalUser.orElse(null);
}
}
```
4. 测试缓存:
```java
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserServiceTest {
@Autowired
private UserService userService;
@Test
public void testGetUserById() {
Long id = 1L;
User user = userService.getUserById(id);
System.out.println(user);
// 再次调用,应该从缓存中获取数据
User cachedUser = userService.getUserById(id);
System.out.println(cachedUser);
}
}
```
在第一次调用getUserById方法时,会从数据库中获取数据并添加到缓存中。在第二次调用时,应该从缓存中获取数据,而不是再次查询数据库。
以上是使用EhCache的示例,如果你想使用其他缓存技术,只需要在依赖中添加相应的库,并在application.properties文件中配置即可。
相关推荐
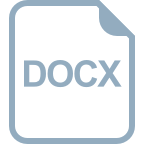
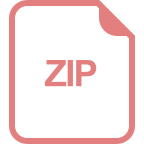
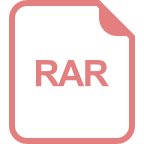














