unity倒计时后开始计时
时间: 2023-05-21 09:07:32 浏览: 231
好的,可以使用 Unity 的 Coroutine 实现倒计时。下面是一段简单的倒计时脚本代码:
```csharp
using UnityEngine;
using System.Collections;
public class Countdown : MonoBehaviour {
public float timeLeft = 60.0f;
void Start() {
StartCoroutine("CountdownTimer");
}
IEnumerator CountdownTimer() {
while (timeLeft > 0) {
yield return new WaitForSeconds(1.0f);
timeLeft--;
Debug.Log("Time Left: " + timeLeft);
}
// do something after countdown finishes
Debug.Log("Countdown Finished!");
yield break;
}
}
```
这里使用了 IEnumerator 和 WaitForSeconds 来实现倒计时功能。注意使用 yield break 来终止协程。
相关问题
Unity 倒计时
在 Unity 中实现倒计时可以通过以下步骤:
1. 创建一个空的 GameObject,给它起一个名字比如 "CountdownTimer"。
2. 在 CountdownTimer 上新增一个 C# 脚本,比如叫做 "CountdownTimer.cs"。
3. 在 CountdownTimer.cs 中,定义一个 public 变量用来存储倒计时的时间,比如叫做 "timeLeft"。
4. 在 Start 方法中初始化 timeLeft 变量,比如设置为 60 秒。
5. 在 Update 方法中,每帧更新 timeLeft 变量,比如通过 Time.deltaTime 函数来减去经过的时间。
6. 在 Update 方法中,检查 timeLeft 是否已经小于等于 0,如果是,就执行倒计时结束后的操作,比如触发游戏失败等。
7. 在 UI 中显示倒计时的剩余时间,可以使用 Text 组件和 CountdownTimer.cs 中的 timeLeft 变量来实现。
下面是一个示例代码:
```
using UnityEngine;
using UnityEngine.UI;
public class CountdownTimer : MonoBehaviour
{
public float timeLeft = 60.0f;
private Text countdownText;
void Start()
{
countdownText = GetComponent<Text>();
}
void Update()
{
timeLeft -= Time.deltaTime;
countdownText.text = "Time Left: " + Mathf.RoundToInt(timeLeft).ToString();
if (timeLeft <= 0)
{
// do something when countdown is finished
}
}
}
```
在上面的示例代码中,我们使用了 Text 组件来显示倒计时的剩余时间,并且在 Update 方法中每帧更新了这个组件的文本内容。同时,在 timeLeft 小于等于 0 时,我们可以执行一些游戏失败的操作。
unity倒计时死亡
在Unity中,创建一个倒计时死亡效果通常用于游戏中的角色生命值系统。以下是简单的步骤:
1. 创建一个空物体作为定时器容器:首先,在场景中创建一个空的游戏对象,并给它命名为"DeathTimer"。
2. 添加时间组件:在该物体上添加`TimeManager`组件,这将负责管理倒计时。
3. 设置生命值:为角色设定一个初始的生命值,并存储在某个变量里,比如`public float health`。
4. 编写脚本:编写一个C#脚本来控制倒计时,当生命值降到0时触发死亡事件。在`Update()`函数中检查生命值,如下面的伪代码所示:
```csharp
public class CountdownDeath : MonoBehaviour
{
public float health;
private float timer;
void Start()
{
timer = health;
}
void Update()
{
timer -= Time.deltaTime;
if (timer <= 0)
{
Die();
}
else
{
// 显示倒计时 UI
GetComponent<Text>().text = "剩余生命:" + Mathf.Round(timer).ToString();
}
}
void Die()
{
// 游戏逻辑,比如播放死亡动画、移除角色等
Destroy(gameObject);
}
}
```
5. 使用UI显示倒计时:在上述代码中,你可以通过`GetComponent<Text>()`获取到一个Text组件来显示剩余的生命值,当倒计时结束时,调用`Die()`方法销毁这个物体。
阅读全文
相关推荐
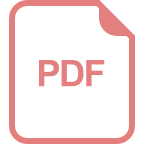
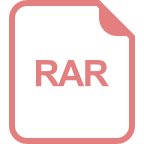
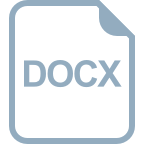










