p ython元组和列表字典举例
时间: 2023-07-30 07:05:21 浏览: 154
好的,下面是 Python 中元组、列表、字典的举例:
1. 元组(tuple):
元组是一种不可变的序列,通常用于存储一些不可变的数据,如坐标、RGB 颜色值等。
```python
# 创建元组
coordinates = (3, 4)
# 访问元组中的元素
x = coordinates[0]
y = coordinates[1]
print(x, y) # 输出:3 4
# 尝试修改元组中的元素,会抛出 TypeError 异常
coordinates[0] = 5 # 抛出 TypeError 异常
```
2. 列表(list):
列表是一种可变的序列,可以存储任意类型的数据,并且支持增删改查等操作。
```python
# 创建列表
fruits = ["apple", "banana", "orange"]
# 访问列表中的元素
first_fruit = fruits[0]
print(first_fruit) # 输出:apple
# 修改列表中的元素
fruits[0] = "pear"
print(fruits) # 输出:['pear', 'banana', 'orange']
# 在列表末尾添加一个元素
fruits.append("grape")
print(fruits) # 输出:['pear', 'banana', 'orange', 'grape']
# 删除列表中的一个元素
del fruits[1]
print(fruits) # 输出:['pear', 'orange', 'grape']
```
3. 字典(dictionary):
字典是一种可变的键值对集合,用于存储具有唯一键的数据,如学生信息、词汇表等。
```python
# 创建字典
student = {"name": "Alice", "age": 20, "major": "Computer Science"}
# 访问字典中的元素
name = student["name"]
print(name) # 输出:Alice
# 修改字典中的元素
student["age"] = 21
print(student) # 输出:{'name': 'Alice', 'age': 21, 'major': 'Computer Science'}
# 添加一个键值对
student["id"] = 1001
print(student) # 输出:{'name': 'Alice', 'age': 21, 'major': 'Computer Science', 'id': 1001}
# 删除一个键值对
del student["major"]
print(student) # 输出:{'name': 'Alice', 'age': 21, 'id': 1001}
```
阅读全文
相关推荐
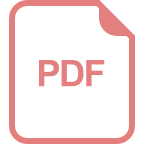
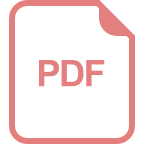













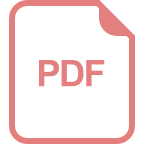
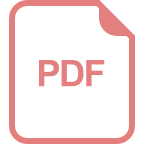
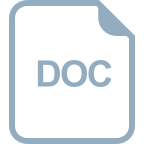