python中进行kmp匹配算法代码
时间: 2023-10-03 12:08:22 浏览: 158
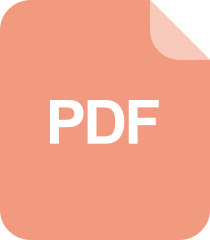
Python实现字符串匹配的KMP算法
Sure! Here's an example code for the KMP algorithm in Python:
```python
def compute_lps(pattern):
lps = [0] * len(pattern)
length = 0
i = 1
while i < len(pattern):
if pattern[i] == pattern[length]:
length += 1
lps[i] = length
i += 1
else:
if length != 0:
length = lps[length - 1]
else:
lps[i] = 0
i += 1
return lps
def kmp(text, pattern):
lps = compute_lps(pattern)
i = j = 0
matches = []
while i < len(text):
if text[i] == pattern[j]:
i += 1
j += 1
if j == len(pattern):
matches.append(i - j)
j = lps[j - 1]
else:
if j != 0:
j = lps[j - 1]
else:
i += 1
return matches
# Example usage
text = "ABCABCDABABCDABCDABDE"
pattern = "ABCDABD"
matches = kmp(text, pattern)
if len(matches) > 0:
print("Pattern found at indices:", matches)
else:
print("Pattern not found.")
```
This code defines two functions: `compute_lps` and `kmp`. The `compute_lps` function is used to compute the Longest Proper Prefix which is also a Suffix (LPS) array for the given pattern. The `kmp` function performs the actual KMP search on the text using the LPS array.
In the example usage, we search for the pattern "ABCDABD" in the text "ABCABCDABABCDABCDABDE". If a match is found, the indices where the pattern occurs in the text will be printed. Otherwise, it will print "Pattern not found."
Note that this is a basic implementation, and you may need to modify it based on your specific requirements.
阅读全文
相关推荐
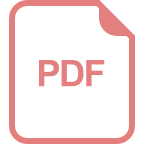
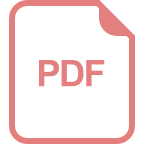
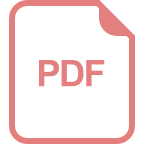
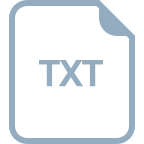
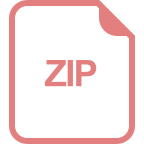
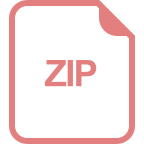
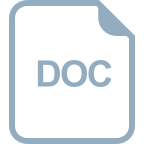
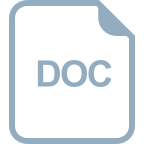







