请完成以下功能的代码 (1)假设每个同学有三门成绩 请随机生成 100 个同学成绩【学号为 9122001--9122100】【成绩为浮点型保留一位小 数,后面相同要求】(成绩要求在 10-100 之间) (2) 将 100 个同学的学号和成绩以文本的形式保存在 文本文件 a.txt 中,每个同学 学号 和 三门成绩一行,成绩之间用逗号分隔。 例如 9122201,88,77,99 9122202,89,74,79 (3) 再次从 a.txt 文件中 读出成绩保存在数组中,打印输出
时间: 2023-11-22 15:53:39 浏览: 40
```python
import random
# 生成100个学生的学号和成绩
scores = []
for i in range(9122001, 9122101):
score = [round(random.uniform(10, 100), 1) for _ in range(3)]
scores.append((i, score))
# 将学号和成绩写入文件
with open('a.txt', 'w') as f:
for s in scores:
f.write(str(s[0]) + ',' + ','.join(map(str, s[1])) + '\n')
# 从文件中读取学号和成绩
with open('a.txt', 'r') as f:
lines = f.readlines()
scores = [(int(line.split(',')[0]), list(map(float, line.split(',')[1:]))) for line in lines]
# 打印学号和成绩
for s in scores:
print(s[0], s[1])
```
相关问题
请完成以下功能的代码 (1)假设每个同学有三门成绩 请随机生成 100 个同学成绩【学号为 9122001--9122100】【成绩为浮点型保留一位小 数,后面相同要求】(成绩要求在 10-100 之间) (2) 将 100 个同学的学号和成绩以文本的形式保存在 文本文件 a.txt 中,每个同学 学号 和 三门成绩一行,成绩之间用逗号分隔。 例如 9122201,88,77,99 9122202,89,74,79 (3) 再次从 a.txt 文件中 读出成绩保存在数组中,打印输
以下是 Python 的代码实现:
```python
import random
# 生成学号
def generate_id():
return str(random.randint(9122001, 9122100))
# 生成成绩
def generate_score():
return round(random.uniform(10, 100), 1)
# 生成所有学生的信息
students = []
for i in range(100):
id = generate_id()
scores = [generate_score() for j in range(3)]
student = (id, scores)
students.append(student)
# 将学生信息写入文件
with open('a.txt', 'w') as f:
for student in students:
line = student[0] + ',' + ','.join(str(score) for score in student[1]) + '\n'
f.write(line)
# 从文件中读取学生信息
with open('a.txt', 'r') as f:
lines = f.readlines()
scores = []
for line in lines:
parts = line.strip().split(',')
id = parts[0]
scores.append((id, [float(score) for score in parts[1:]]))
# 打印成绩
for score in scores:
print(score)
```
以上代码随机生成了 100 个学生的成绩并保存在文件中,然后再从文件中读取成绩并打印输出。
请完成以下C语言功能的代码 (1)假设每个同学有三门成绩 请随机生成 100 个同学成绩【学号为 9122001--9122100】【成绩为浮点型保留一位小 数,后面相同要求】(成绩要求在 10-100 之间) (2) 将 100 个同学的学号和成绩以文本的形式保存在 文本文件 a.txt 中,每个同学 学号 和 三门成绩一行,成绩之间用逗号分隔。 例如 9122201,88,77,99 9122202,89,74,79 (3) 再次从 a.txt 文件中 读出成绩保存在数组中,打印输
以下是 C 语言的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define NUM_STUDENTS 100
#define ID_START 9122001
#define ID_END 9122100
#define SCORE_MIN 10.0
#define SCORE_MAX 100.0
int main() {
// 生成学生信息
srand(time(NULL));
float students[NUM_STUDENTS][4];
for (int i = 0; i < NUM_STUDENTS; i++) {
float id = ID_START + i;
float score1 = (float)(rand()) / RAND_MAX * (SCORE_MAX - SCORE_MIN) + SCORE_MIN;
float score2 = (float)(rand()) / RAND_MAX * (SCORE_MAX - SCORE_MIN) + SCORE_MIN;
float score3 = (float)(rand()) / RAND_MAX * (SCORE_MAX - SCORE_MIN) + SCORE_MIN;
students[i][0] = id;
students[i][1] = score1;
students[i][2] = score2;
students[i][3] = score3;
}
// 将学生信息写入文件
FILE *fp = fopen("a.txt", "w");
for (int i = 0; i < NUM_STUDENTS; i++) {
fprintf(fp, "%.0f,%.1f,%.1f,%.1f\n", students[i][0], students[i][1], students[i][2], students[i][3]);
}
fclose(fp);
// 从文件中读取学生信息
fp = fopen("a.txt", "r");
float scores[NUM_STUDENTS][4];
for (int i = 0; i < NUM_STUDENTS; i++) {
fscanf(fp, "%f,%f,%f,%f\n", &scores[i][0], &scores[i][1], &scores[i][2], &scores[i][3]);
}
fclose(fp);
// 打印成绩
for (int i = 0; i < NUM_STUDENTS; i++) {
printf("%.0f,%.1f,%.1f,%.1f\n", scores[i][0], scores[i][1], scores[i][2], scores[i][3]);
}
return 0;
}
```
以上代码随机生成了 100 个学生的成绩并保存在文件中,然后再从文件中读取成绩并打印输出。
相关推荐
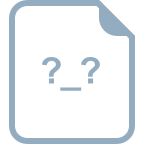
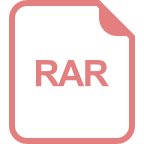
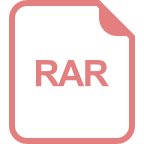













