boxes, confidences, class_ids = model.predict(image) ,model是什么意思
时间: 2024-06-08 19:05:33 浏览: 7
这句代码中的`model`是一个机器学习模型,用于对输入的图像进行目标检测。在深度学习中,训练模型需要大量的数据和计算资源,并通过反向传播算法来优化模型参数,从而使模型能够准确地预测新的数据。在本句代码中,`model.predict()`方法用于对输入的图像进行预测,并返回预测结果,其中包括检测到的物体框、置信度和类别ID等信息。
相关问题
解释代码: def detect_cma(self): # pass model = self.model output_size = self.output_size # source = self.img2predict # file/dir/URL/glob, 0 for webcam imgsz = [640, 640] # inference size (pixels) conf_thres = 0.25 # confidence threshold iou_thres = 0.45 # NMS IOU threshold max_det = 1000 # maximum detections per image # device = self.device # cuda device, i.e. 0 or 0,1,2,3 or cpu view_img = False # show results save_txt = False # save results to *.txt save_conf = False # save confidences in --save-txt labels save_crop = False # save cropped prediction boxes nosave = False # do not save images/videos classes = None # filter by class: --class 0, or --class 0 2 3 agnostic_nms = False # class-agnostic NMS augment = False # ugmented inference visualize = False # visualize features line_thickness = 3 # bounding box thickness (pixels) hide_labels = False # hide labels hide_conf = False # hide confidences half = False # use FP16 half-precision inference dnn = False # use OpenCV DNN for ONNX inference source = str(self.vid_source) device = select_device(self.device) stride, names, pt, jit, onnx = model.stride, model.names, model.pt, model.jit, model.onnx imgsz = check_img_size(imgsz, s=stride) # check image size save_img = not nosave and not source.endswith('.txt') # save inference images
这段代码用于使用 YOLOv5 模型对图像或视频进行目标检测。它首先从类的属性中获取模型、输出大小、视频源等信息。然后设置了一些参数,如推理尺寸、置信度阈值、NMS IOU 阈值等。接着根据设备类型选择使用 CPU 还是 GPU 进行推理。最后,它检查图像的大小是否符合模型要求,设置是否保存推理结果图片,然后返回检测结果。
import pandas data=pandas.read_csv('D:/py_anli2/apriori.csv',encoding='GBK') # print(data) transactions= data['items'].values from apyori import apriori results = list( apriori( transactions, min_support=0.001, min_confidence=0.001, min_lift=1.001 ) ) supports=[] confidences=[] lifts=[] bases=[] adds=[] for r in results: size = len(r.ordered_statistics) for j in range(size): supports.append(r.support) confidences.append( r.ordered_statistics[j].confidence ) lifts.append(r.ordered_statistics[j].lift) bases.append( list(r.ordered_statistics[j].items_base) ) adds.append( list(r.ordered_statistics[j].items_add) ) result = pandas.DataFrame({ '基于':bases, '推荐':adds, '支持度':supports, '置信度':confidences, '提升度':lifts, }) print(result)
这段代码是使用Apriori算法进行关联规则挖掘分析。它使用pandas库读取一个CSV文件,然后从文件中提取出需要进行关联规则挖掘的事务数据。接下来,它使用apyori库中的apriori函数来执行关联规则挖掘,并将结果存储在一个列表中。然后,通过遍历结果列表,将每个关联规则的支持度、置信度、提升度、基于项和推荐项存储在各自的列表中。最后,将这些列表整理成一个pandas DataFrame,并打印输出结果。
需要注意的是,这段代码中的文件路径可能需要根据实际情况进行修改。另外,关联规则挖掘的参数(如最小支持度、最小置信度、最小提升度)也可以根据具体需求进行调整。
相关推荐
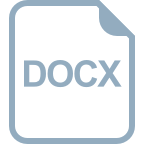
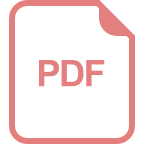
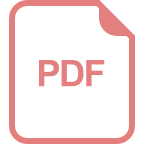













