opencv python 假设将图像分离为RGB三个通道 对每个通道进行递归迭代,如何找到矩形外框并得到相应的分割阈值
时间: 2023-11-27 13:52:31 浏览: 75
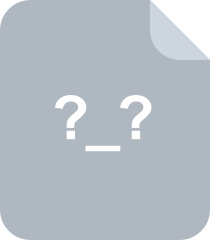
图像分割迭代法求阈值

可以使用以下步骤找到每个通道的矩形外框并得到相应的分割阈值:
1. 将图像分离为RGB三个通道,可以使用OpenCV中的split函数。
2. 对于每个通道,使用递归函数来进行分割。在递归函数中,首先计算当前图像的直方图,并获取直方图中出现频率最高的像素值及其对应的像素数量。
3. 根据直方图中出现频率最高的像素值,使用threshold函数来将当前图像分割成两个部分。分别计算这两个部分的矩形外框。
4. 如果当前矩形外框的大小小于某个阈值,则停止递归,否则对每个矩形继续递归进行分割。
5. 在递归过程中,记录每个矩形外框的大小及对应的分割阈值。最终选择矩形外框最大的那个作为最终的分割结果。
下面是一个简单的示例代码,可以作为参考:
```
import cv2
import numpy as np
def get_rect(img):
h, w = img.shape[:2]
mask = np.zeros((h+2, w+2), np.uint8)
_, _, rect, _ = cv2.floodFill(img, mask, (0, 0), 255)
return rect
def get_threshold(img):
hist = cv2.calcHist([img], [0], None, [256], [0, 256])
max_val = np.argmax(hist)
_, thresh = cv2.threshold(img, max_val, 255, cv2.THRESH_BINARY)
return get_rect(thresh), max_val
def recursive_split(img):
h, w = img.shape[:2]
if h < 10 or w < 10:
return None
rects = []
thresholds = []
for i in range(3):
channel = img[:,:,i]
rect, threshold = get_threshold(channel)
rects.append(rect)
thresholds.append(threshold)
max_rect = max(rects, key=lambda x: x[2]*x[3])
max_index = rects.index(max_rect)
threshold = thresholds[max_index]
_, max_thresh = cv2.threshold(img[:,:,max_index], threshold, 255, cv2.THRESH_BINARY)
sub_rects = [recursive_split(max_thresh[rect[1]:rect[1]+rect[3], rect[0]:rect[0]+rect[2]]) for rect in rects]
return (max_thresh, rects[max_index], threshold, sub_rects)
img = cv2.imread('test.jpg')
result = recursive_split(img)
cv2.imshow('result', result[0])
cv2.waitKey(0)
```
阅读全文
相关推荐
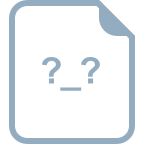


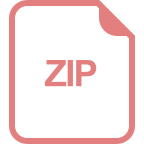

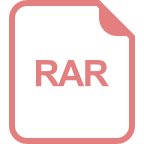
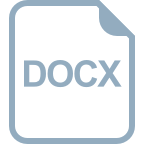
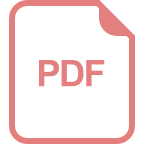
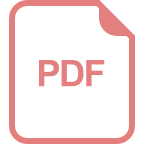
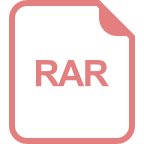
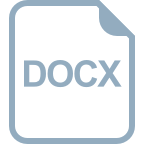
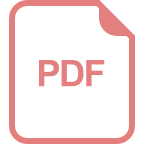
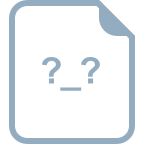
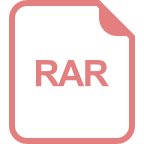
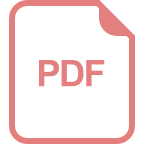
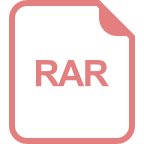
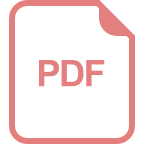