编写一个java测试程序。封装一个 Teacher 类负责给出算术题目,随机给出两个整数并进行运算,判断回答者的答案是否正确;封装一个GUI类 ComputerFrame,回答者可以通过 GU看到题目并给出答案;封装一个程序执行入口类运行该程序。
时间: 2024-12-02 08:20:32 浏览: 15
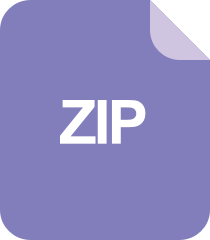
基于Java实现四则运算题目生成程序.zip
编写一个Java测试程序,我们可以按照以下步骤分步创建:
1. **Teacher 类**:
```java
import java.util.Random;
public class Teacher {
private int num1;
private int num2;
public Teacher() {
Random rand = new Random();
this.num1 = rand.nextInt(50); // 生成0到49之间的随机整数
this.num2 = rand.nextInt(50);
}
public String generateQuestion() {
return "请问 " + num1 + " 加上 " + num2 + " 等于多少?";
}
public boolean checkAnswer(int answer) {
return num1 + num2 == answer;
}
}
```
在这个类中,我们定义了生成随机算术题目的方法,并有检查答案是否正确的功能。
2. **ComputerFrame GUI 类** (使用Swing库):
```java
import javax.swing.*;
import java.awt.*;
public class ComputerFrame extends JFrame {
private JTextField answerField;
private JButton submitButton;
private Teacher teacher;
public ComputerFrame(Teacher teacher) {
this.teacher = teacher;
setTitle("数学小测验");
setSize(300, 150);
JPanel panel = new JPanel(new GridLayout(2, 2));
JLabel questionLabel = new JLabel(teacher.generateQuestion());
answerField = new JTextField(10);
submitButton = new JButton("提交");
panel.add(questionLabel);
panel.add(answerField);
panel.add(submitButton);
add(panel);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == submitButton) {
try {
int userAnswer = Integer.parseInt(answerField.getText());
boolean isCorrect = teacher.checkAnswer(userAnswer);
JOptionPane.showMessageDialog(this, isCorrect ? "答对了!" : "答错了!");
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "请输入一个整数!");
}
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new ComputerFrame(new Teacher()).setVisible(true));
}
}
```
这个类包含了用户界面元素,包括显示问题、输入答案的文本框以及提交按钮。当用户提交答案时,会验证其正确性。
3. **程序执行入口类** (Main Class):
```java
public class Main {
public static void main(String[] args) {
new ComputerFrame(new Teacher()).setVisible(true);
}
}
```
这是整个程序的起点,启动`ComputerFrame`的GUI。
阅读全文
相关推荐
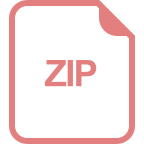
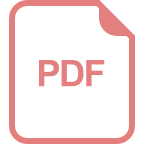
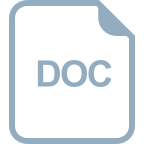
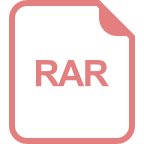
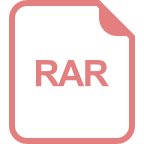
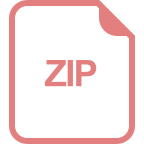
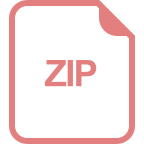
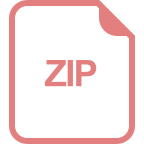
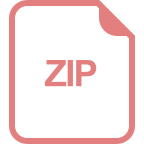
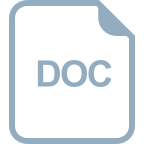
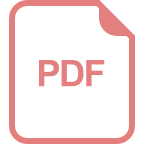
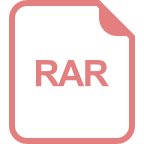
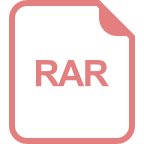
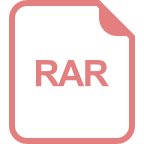
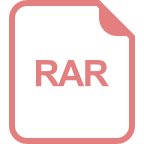
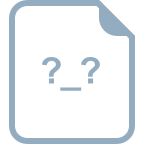